Java: Find the second largest element in an array
Java Array: Exercise-17 with Solution
Write a Java program to find the second largest element in an array.
Pictorial Presentation:
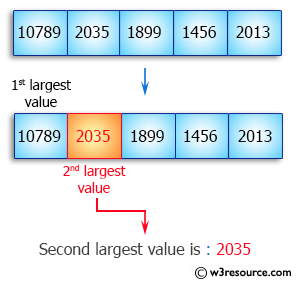
Sample Solution:
Java Code :
// Import the necessary Java utilities package.
import java.util.Arrays;
// Define a class named Main.
public class Main {
// The main method where the program execution starts.
public static void main(String[] args) {
// Create an integer array with numeric values.
int[] my_array = {
10789, 2035, 1899, 1456, 2013,
1458, 2458, 1254, 1472, 2365,
1456, 2165, 1457, 2456};
// Print the original numeric array.
System.out.println("Original numeric array : " + Arrays.toString(my_array));
// Sort the array to find the second largest value.
Arrays.sort(my_array);
// Initialize an index to the last element's index.
int index = my_array.length - 1;
// Find the second largest value by iterating from the end of the array.
while (my_array[index] == my_array[my_array.length - 1]) {
index--;
}
// Print the second largest value found.
System.out.println("Second largest value: " + my_array[index]);
}
}
Sample Output:
Original numeric array : [10789, 2035, 1899, 1456, 2013, 1458, 2458, 1254, 1472, 2365, 1456, 2165, 1457, 2456] Second largest value: 2458
Flowchart:
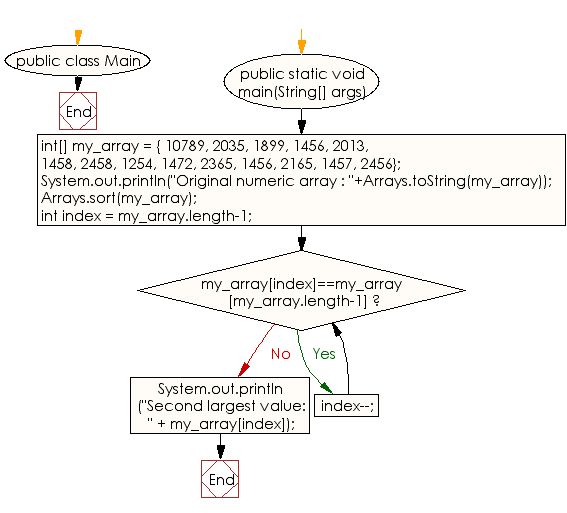
Java Code Editor:
Previous: Write a Java program to find the second smallest element in an array.
Next: Write a Java program to find the second largest element in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics