Java: Count the number of possible triangles from a given unsorted array of positive integers
Java Array: Exercise-44 with Solution
Write a Java program to count the number of possible triangles from a given unsorted array of positive integers.
Note: The triangle inequality states that the sum of the lengths of any two sides of a triangle must be greater than or equal to the length of the third side.
Sample Solution:
Java Code:
// Import necessary Java libraries.
import java.util.*;
import java.lang.*;
// Define a class named Main.
public class Main
{
// The main method for executing the program.
public static void main(String[] args)
{
// Define an array of integers.
int nums[] = {6, 7, 9, 16, 25, 12, 30, 40};
int n = nums.length;
System.out.println("Original Array : " + Arrays.toString(nums));
// Sort the array elements in non-decreasing order.
Arrays.sort(nums);
// Initialize count of triangles.
int ctr = 0;
// Iterate through the array elements to count the number of triangles.
for (int i = 0; i < n - 2; ++i)
{
int x = i + 2;
for (int j = i + 1; j < n; ++j)
{
while (x < n && nums[i] + nums[j] > nums[x])
++x;
ctr += x - j - 1;
}
}
System.out.println("Total number of triangles: " + ctr);
}
}
Sample Output:
Original Array : [6, 7, 9, 16, 25, 12, 30, 40] Total number of triangles: 17
Flowchart:
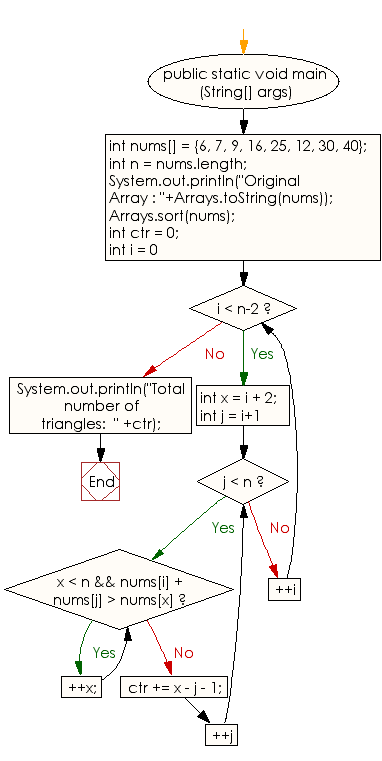
Java Code Editor:
Previous: Write a Java program to find all combination of four elements of a given array whose sum is equal to a given value.
Next: Write a Java program to cyclically rotate a given array clockwise by one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/array/java-array-exercise-44.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics