Java: Shuffle a given array of integers
Java Array: Exercise-60 with Solution
Write a Java program to shuffle a given array of integers.
Example:
Input :
nums = { 1, 2, 3, 4, 5, 6 }
Output:
Shuffle Array: [4, 2, 6, 5, 1, 3]
Sample Solution:
Java Code:
// Import necessary Java classes.
import java.util.Arrays;
import java.util.Random;
// Define a class named 'solution'.
class solution
{
// A method to shuffle an array using the Fisher-Yates algorithm.
public static void shuffle(int nums[])
{
for (int i = nums.length - 1; i >= 1; i--)
{
// Create a random number generator.
Random rand = new Random();
// Generate a random index j between 0 and i (inclusive).
int j = rand.nextInt(i + 1);
// Swap elements at indices i and j.
swap_elements(nums, i, j);
}
}
// A method to swap two elements in an array.
private static void swap_elements(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
public static void main (String[] args)
{
int[] nums = { 1, 2, 3, 4, 5, 6 };
System.out.println("Original Array: "+Arrays.toString(nums));
// Shuffle the array using the Fisher-Yates algorithm.
shuffle(nums);
System.out.println("Shuffled Array: "+Arrays.toString(nums));
}
}
Sample Output:
Original Array: [1, 2, 3, 4, 5, 6] Shuffle Array: [4, 2, 6, 5, 1, 3]
Flowchart:
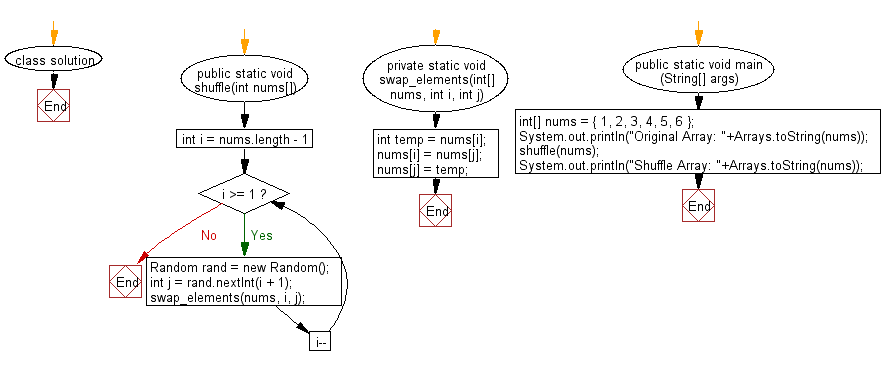
Java Code Editor:
Previous: Write a Java program to find maximum product of two integers in a given array of integers.
Next: Write a Java program to rearrange a given array of unique elements such that every second element of the array is greater than its left and right elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/array/java-array-exercise-60.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics