Java: Rearrange a given array of unique elements such that every second element of the array is greater than its left and right elements
Java Array: Exercise-61 with Solution
Write a Java program to rearrange a given array of unique elements such that every second element of the array is greater than its left and right elements.
Example:
Input :
nums= { 1, 2, 4, 9, 5, 3, 8, 7, 10, 12, 14 }
Output:
Array with every second element is greater than its left and right elements:
[1, 4, 2, 9, 3, 8, 5, 10, 7, 14, 12]
Sample Solution:
Java Code:
// Import the necessary Java class.
import java.util.Arrays;
// Define a class named 'solution'.
class solution
{
// A private method to swap two elements in an array.
private static void swap_nums(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
// A method to rearrange an array such that every second element is greater
// than its left and right elements.
public static void rearrange_Array_nums(int[] nums)
{
for (int i = 1; i < nums.length; i += 2)
{
// Check if the element to the left is greater and swap if needed.
if (nums[i - 1] > nums[i]) {
swap_nums(nums, i - 1, i);
}
// Check if the element to the right is greater and swap if needed.
if (i + 1 < nums.length && nums[i + 1] > nums[i]) {
swap_nums(nums, i + 1, i);
}
}
}
public static void main (String[] args)
{
int[] nums= { 1, 2, 4, 9, 5, 3, 8, 7, 10, 12, 14 };
System.out.println("Original array:\n"+Arrays.toString(nums));
// Rearrange the array so that every second element is greater than its
// left and right elements.
rearrange_Array_nums(nums);
System.out.println("\nArray with every second element is greater than its left and right elements:\n"
+ Arrays.toString(nums));
}
}
Sample Output:
Original array: [1, 2, 4, 9, 5, 3, 8, 7, 10, 12, 14] Array with every second element is greater than its left and right elements: [1, 4, 2, 9, 3, 8, 5, 10, 7, 14, 12]
Flowchart:
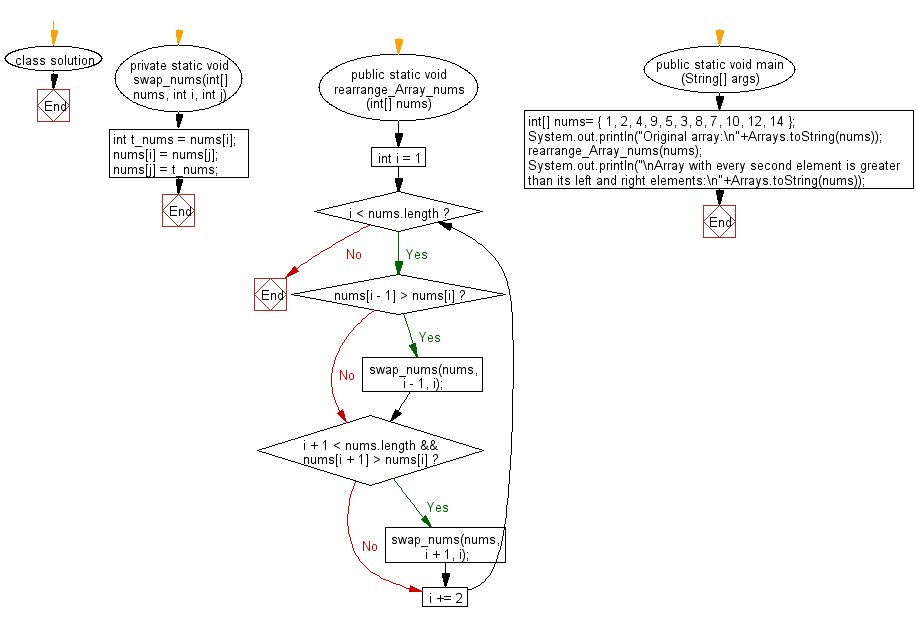
Java Code Editor:
Previous: Write a Java program to shuffle a given array of integers.
Next: Write a Java program to find the equilibrium indices from a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/array/java-array-exercise-61.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics