Java: Form the largest number from a given list of non negative integers
Java Array: Exercise-71 with Solution
Write a Java program to find the largest number from a given list of non-negative integers.
Example:
Input :
nums = {1, 2, 3, 0, 4, 6}
Output:
Largest number using the said array numbers: 643210
Sample Solution:
Java Code:
// Import the necessary Java classes.
import java.util.*;
// Define the 'solution' class.
public class solution {
// Define a method to find the largest number using an array of numbers.
public static String largest_Numbers(int[] num) {
// Convert the array of numbers to an array of strings.
String[] array_nums = Arrays.stream(num).mapToObj(String::valueOf).toArray(String[]::new);
// Sort the array of strings in descending order, considering their concatenated values.
Arrays.sort(array_nums, (String str1, String str2) -> (str2 + str1).compareTo(str1 + str2));
// Reduce the sorted array to find the largest number.
return Arrays.stream(array_nums).reduce((a, b) -> a.equals("0") ? b : a + b).get();
}
public static void main(String[] args)
{
// Initialize an array of numbers.
int[] nums = {1, 2, 3, 0, 4, 6};
// Print the original array and the largest number using the array elements.
System.out.printf("\nOriginal array: " + Arrays.toString(nums));
System.out.printf("\nLargest number using the said array numbers: " + largest_Numbers(nums));
}
}
Sample Output:
Original array: [1, 2, 3, 0, 4, 6] Largest number using the said array numbers: 643210
Flowchart:
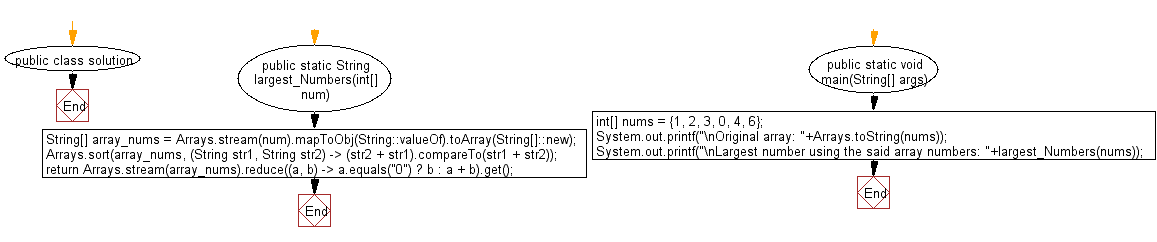
Java Code Editor:
Previous: Write a Java program to find the smallest length of a contiguous subarray of which the sum is greater than or equal to specified value. Return 0 instead.
Next: Write a Java program to find and print one continuous subarray (from a given array of integers) that if you only sort the said subarray in ascending order then the entire array will be sorted in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/array/java-array-exercise-71.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics