Java: Create a new string taking first three characters from a given string
Java Basic: Exercise-72 with Solution
First 3 Chars or #
Write a Java program to create a string taking the first three characters from a given string. If the string length is less than 3 use "#" as substitute characters.
Test Data: str1 = "Python"
str2 = " "
Sample Solution:
Java Code:
import java.lang.*;
public class Exercise72 {
public static void main(String[] args) {
// Define an empty string
String str1 = "";
// Get the length of the string
int len = str1.length();
// Check the length of the string and take different actions based on its length
if (len >= 3) {
// If the string has three or more characters, print the first three characters
System.out.println(str1.substring(0, 3));
} else if (len == 1) {
// If the string has only one character, add "##" to it and print
System.out.println(str1.charAt(0) + "##");
} else {
// If the string is empty or has two characters, print "###"
System.out.println("###");
}
}
}
Sample Output:
###
Pictorial Presentation:
Flowchart:
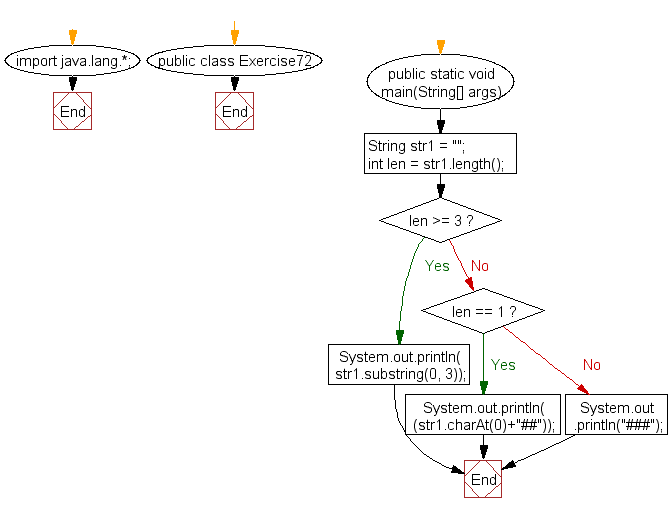
Java Code Editor:
Previous: Write a Java program to create the concatenation of the two strings except removing the first character of each string.
Next: Write a Java program to create a new string taking first and last characters from two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/basic/java-basic-exercise-72.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics