Java: Find the numbers less than 7 in a tree set
9. Elements Less Than 7 in TreeSet
Write a Java program to find numbers less than 7 in a tree set.
Sample Solution:
Java Code:
import java.util.TreeSet;
import java.util.Iterator;
public class Exercise9 {
public static void main(String[] args) {
// creating TreeSet
TreeSet <Integer>tree_num = new TreeSet<Integer>();
TreeSet <Integer>treeheadset = new TreeSet<Integer>();
// Add numbers in the tree
tree_num.add(1);
tree_num.add(2);
tree_num.add(3);
tree_num.add(5);
tree_num.add(6);
tree_num.add(7);
tree_num.add(8);
tree_num.add(9);
tree_num.add(10);
// Find numbers less than 7
treeheadset = (TreeSet)tree_num.headSet(7);
// create an iterator
Iterator iterator;
iterator = treeheadset.iterator();
//Displaying the tree set data
System.out.println("Tree set data: ");
while (iterator.hasNext()){
System.out.println(iterator.next() + " ");
}
}
}
Sample Output:
Note: Exercise9.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. Tree set data: 1 2 3 5 6
Flowchart:
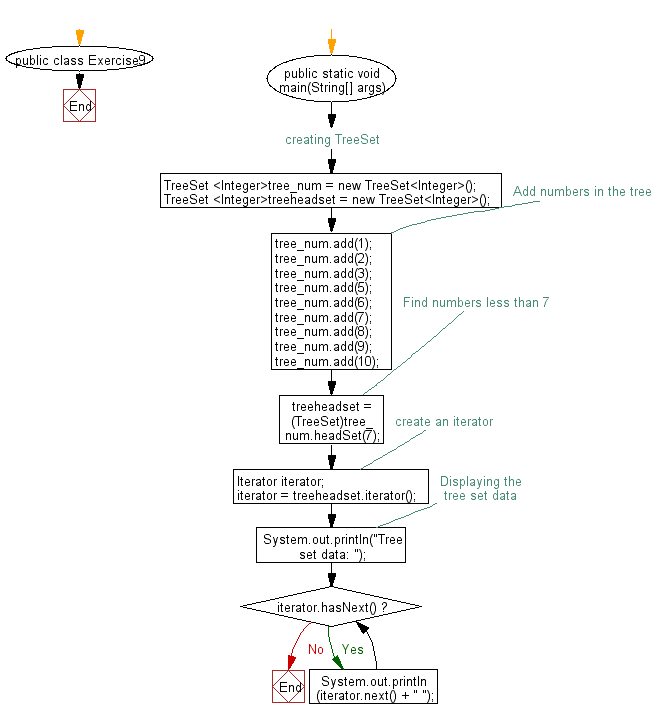
For more Practice: Solve these Related Problems:
- Write a Java program to use the headSet() method on a TreeSet to extract all numbers less than 7 and print the resulting set.
- Write a Java program to filter a TreeSet using Java streams to collect numbers less than 7 into a new set.
- Write a Java program to iterate over a TreeSet and print only those numbers that are less than 7.
- Write a Java program to remove all elements greater than or equal to 7 from a TreeSet and then display the remaining elements.
Go to:
PREV : Compare TreeSets.
NEXT : TreeSet Ceiling Element.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.