Java: Display the following character rhombus structure
Java Conditional Statement: Exercise-26 with Solution
Character Rhombus Structure
Write a Java program to display the following character rhombus structure.
Test Data
Input the number: 7
Pictorial Presentation:
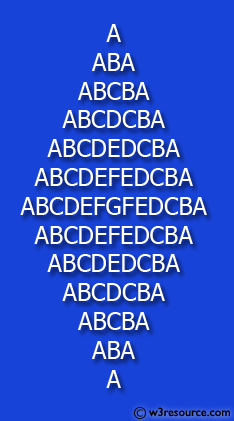
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise26 {
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.println("Input the number: ");
int n = sc.nextInt();
int count = 1;
int count2 = 1;
char c = 'A';
for (int i = 1; i < (n * 2); i++)
{
for (int spc = n - count2; spc > 0; spc--)
//print space
{
System.out.print(" ");
}
if (i < n)
{
count2++;
}
else
{
count2--;
}
for (int j = 0; j < count; j++)
{
System.out.print(c);//print Character
if (j < count / 2)
{
c++;
} else
{
c--;
}
}
if (i < n)
{
count = count + 2;
}
else
{
count = count - 2;
}
c = 'A';
System.out.println();
}
}
}
Sample Output:
Input the number: 7 A ABA ABCBA ABCDCBA ABCDEDCBA ABCDEFEDCBA ABCDEFGFEDCBA ABCDEFEDCBA ABCDEDCBA ABCDCBA ABCBA ABA A
Flowchart:
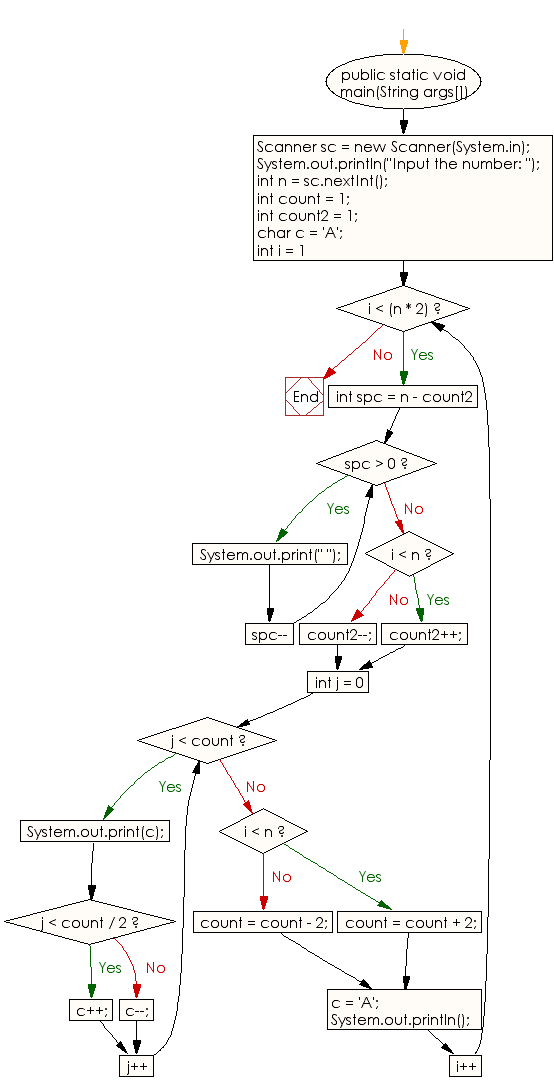
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a java program to display the number rhombus structure.
Next: Write a Java program that reads an integer and check whether it is negative, zero, or positive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/conditional-statement/java-conditional-statement-exercise-26.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics