Java Encapsulation: Implementing Person Class with Getter and Setter Methods
Java Encapsulation: Exercise-1 with Solution
Write a Java program to create a class called Person with private instance variables name, age. and country. Provide public getter and setter methods to access and modify these variables.
Sample Solution:
Java Code:
// Person.java
// Person Class
class Person {
private String name;
private int age;
private String country;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
}
// Main.java
// Main Class
public class Main {
public static void main(String[] args) {
// Create an instance of Person
Person person = new Person();
// Set values using setter methods
person.setName("Arthfael Viktorija");
person.setAge(25);
person.setCountry("USA");
// Get values using getter methods
String name = person.getName();
int age = person.getAge();
String country = person.getCountry();
// Print the values
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("Country: " + country);
}
}
Sample Output:
Name: Arthfael Viktorija Age: 25 Country: US
Explanation:
In the above exercise, the Person class encapsulates the private instance variables name, age, and country. These variables are declared private, which means they cannot be accessed outside the Person class.
To access and modify these private variables, public getter and setter methods are provided. The getter methods (getName(), getAge(), and getCountry()) allow other classes to retrieve the values of private variables. The setter methods (setName(), setAge(), and setCountry()) provide a way to modify the values of private variables.
In the Main class, an instance of the Person class is created using the Person constructor (Person person = new Person()). Then, the setter methods are used to set the values of the private variables name, age, and country.
To retrieve these values, the getter methods are called (String name = person.getName(), int age = person.getAge(), String country = person.getCountry()). Finally, the retrieved values are printed using System.out.println().
Flowchart:
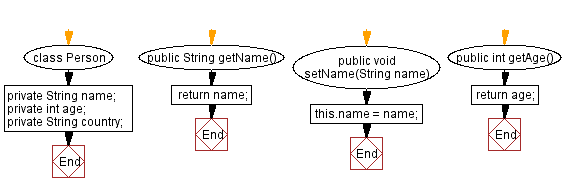
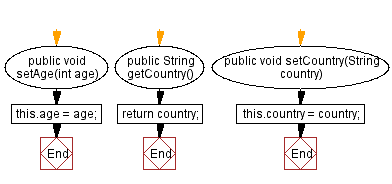
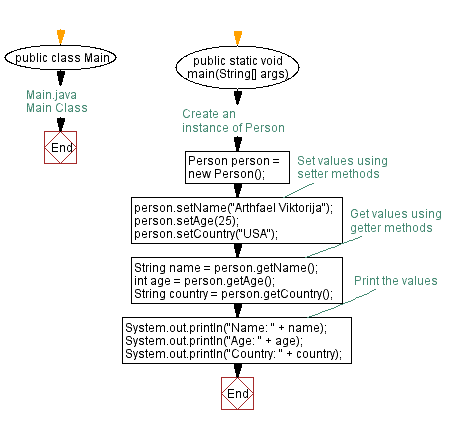
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java Encapsulation Exercises Home.
Next: Implementing the BankAccount Class with Getter and Setter Methods.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics