Java Encapsulation: Implementing a circle class with getter, setter, and calculation methods
Java Encapsulatiion: Exercise-5 with Solution
Write a Java program to create a class called Circle with a private instance variable radius. Provide public getter and setter methods to access and modify the radius variable. However, provide two methods called calculateArea() and calculatePerimeter() that return the calculated area and perimeter based on the current radius value.
Sample Solution:
Java Code:
// Circle.java
// Circle Class
class Circle {
// Declare a private double variable for radius
private double radius;
// Getter method for radius
public double getRadius() {
return radius;
}
// Setter method for radius
public void setRadius(double radius) {
this.radius = radius;
}
// Method to calculate the area of the circle
public double calculateArea() {
return Math.PI * radius * radius;
}
// Method to calculate the perimeter (circumference) of the circle
public double calculatePerimeter() {
return 2 * Math.PI * radius;
}
}
// Main.java
// Main Class
public class Main {
public static void main(String[] args) {
// Create an instance of Circle
Circle circle = new Circle();
// Set the radius using the setter method
circle.setRadius(7.0);
// Get the radius using the getter method
double radius = circle.getRadius();
// Calculate and print the area and perimeter
double area = circle.calculateArea();
double perimeter = circle.calculatePerimeter();
System.out.println("Circle Radius: " + radius);
System.out.println("Circle Area: " + area);
System.out.println("Circle Perimeter: " + perimeter);
}
}
Output:
Circle Radius: 7.0 Circle Area: 153.93804002589985 Circle Perimeter: 43.982297150257104
Explanation:
In the above exercise,
In this code, the Circle class encapsulates the private instance variable radius. The getRadius() and setRadius() methods are public getter and setter methods that allow other classes to access and modify radius values, respectively.
Additionally, the Circle class provides two public methods calculateArea() and calculatePerimeter() that calculate and return the area and perimeter of the circle. These methods are based on the current radius value. These methods use the formula Math.PI*radius*radius for area calculation and 2*Math.PI*radius for perimeter calculation.
In the Main class, an object circle of the Circle class is created. The circle radius is set using the setRadius() method, and then retrieved using the getRadius() method.
The area and perimeter of the circle are calculated using the calculateArea() and calculatePerimeter() methods, respectively, and printed using System.out.println().
Flowchart:
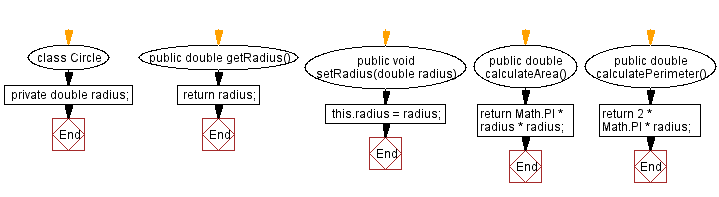
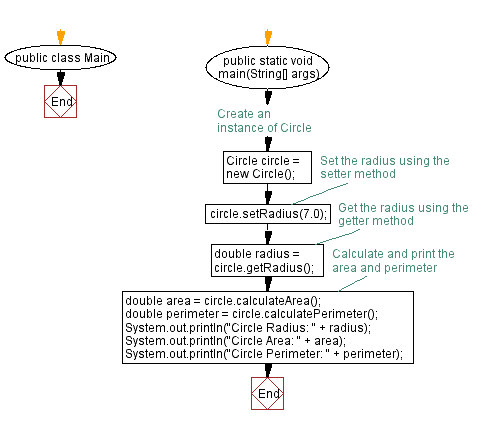
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Implementing an Employee Class with Getter and Setter Methods.
Next: Implementing Car Class with Getter and Setter Methods.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/encapsulation/java-encapsulation-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics