Java Encapsulation: Implementing a Student Class with Grade Validation
Java Encapsulatiion: Exercise-7 with Solution
Write a Java program to create a class called Student with private instance variables student_id, student_name, and grades. Provide public getter and setter methods to access and modify the student_id and student_name variables. However, provide a method called addGrade() that allows adding a grade to the grades variable while performing additional validation.
Sample Solution:
Java Code:
Student.java
// Import the ArrayList and List classes from the java.util package
import java.util.ArrayList;
import java.util.List;
// Student Class
class Student {
// Declare a private int variable for the student ID
private int student_id;
// Declare a private String variable for the student name
private String student_name;
// Declare a private List of Double for the grades
private List grades;
// Getter method for student_id
public int getStudent_id() {
return student_id;
}
// Setter method for student_id
public void setStudent_id(int student_id) {
this.student_id = student_id;
}
// Getter method for student_name
public String getStudent_name() {
return student_name;
}
// Setter method for student_name
public void setStudent_name(String student_name) {
this.student_name = student_name;
}
// Getter method for grades
public List getGrades() {
return grades;
}
// Method to add a grade to the grades list
public void addGrade(double grade) {
// Initialize the grades list if it is null
if (grades == null) {
grades = new ArrayList<>();
}
// Add the grade to the grades list
grades.add(grade);
}
}
Main.java|
// Main Class
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create an instance of Student
Student student = new Student();
// Set the values using the setter methods
student.setStudent_id(1);
student.setStudent_name("Nadia Hyakinthos");
// Add grades using the addGrade() method
student.addGrade(92.5);
student.addGrade(89.0);
student.addGrade(90.3);
// Get the values using the getter methods
int student_id = student.getStudent_id();
String student_name = student.getStudent_name();
List<Double> grades = student.getGrades();
// Print the values
System.out.println("Student ID: " + student_id);
System.out.println("Student Name: " + student_name);
System.out.println("Grades: " + grades);
}
}
Output:
Student ID: 1 Student Name: Nadia Hyakinthos Grades: [92.5, 89.0, 90.3]
Explanation:
In the above exercise,
The Student class:
- It defines a class called Student with private instance variables student_id, student_name, and grades.
- The variables are marked as private, which means they can only be accessed within the Student class.
- Public getter and setter methods are provided for student_id and student_name to access and modify these variables.
- Additionally, a getter method is provided for the grades variable, which returns a list of double values.
- There is also a method called addGrade() that allows adding grades to the grades list. It performs additional validation by checking if the grades list is null and initializing it if necessary.
The Main class:
- It contains the main method, which serves as the entry point for the program.
- An instance of the Student class is created using the new keyword.
- The values of student_id and student_name are set using the setter methods.
- Grades are added to the grades list using the addGrade() method.
- The values of student_id, student_name, and grades are retrieved using the respective getter methods.
Flowchart:
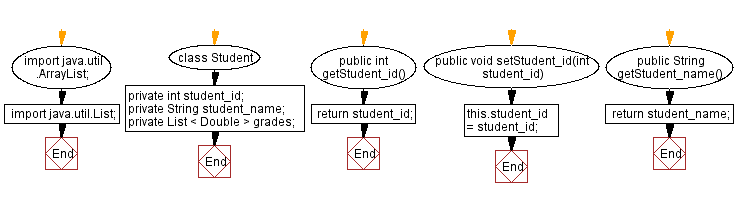
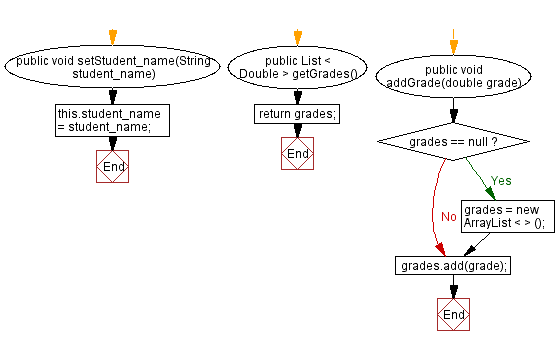
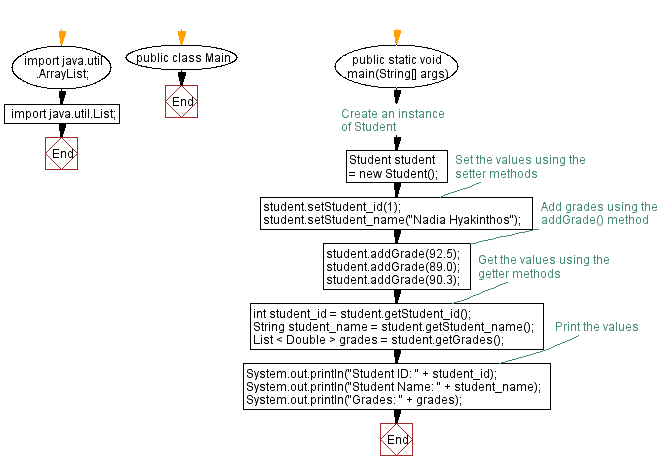
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Implementing Car Class with Getter and Setter Methods.
Next: Creating a Java Book Class with Encapsulation and Discount Method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/encapsulation/java-encapsulation-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics