Java Inheritance Programming - BankAccount class with methods called deposit() and withdraw()
Write a Java program to create a class known as "BankAccount" with methods called deposit() and withdraw(). Create a subclass called SavingsAccount that overrides the withdraw() method to prevent withdrawals if the account balance falls below one hundred.
Sample Solution:
Java Code:
// BankAccount.java
// Parent class BankAccount
// Declare the BankAccount class
public class BankAccount {
// Private field to store the account number
private String accountNumber;
// Private field to store the balance
private double balance;
// Constructor to initialize account number and balance
public BankAccount(String accountNumber, double balance) {
this.accountNumber = accountNumber;
this.balance = balance;
}
// Method to deposit an amount into the account
public void deposit(double amount) {
// Increase the balance by the deposit amount
balance += amount;
}
// Method to withdraw an amount from the account
public void withdraw(double amount) {
// Check if the balance is sufficient for the withdrawal
if (balance >= amount) {
// Decrease the balance by the withdrawal amount
balance -= amount;
} else {
// Print a message if the balance is insufficient
System.out.println("Insufficient balance");
}
}
// Method to get the current balance
public double getBalance() {
// Return the current balance
return balance;
}
}
// SavingsAccount.java
// Child class SavingsAccount
// Declare the SavingsAccount class, inheriting from BankAccount
public class SavingsAccount extends BankAccount {
// Constructor to initialize account number and balance
public SavingsAccount(String accountNumber, double balance) {
// Call the parent class constructor
super(accountNumber, balance);
}
// Override the withdraw method from the parent class
@Override
public void withdraw(double amount) {
// Check if the withdrawal would cause the balance to drop below $100
if (getBalance() - amount < 100) {
// Print a message if the minimum balance requirement is not met
System.out.println("Minimum balance of $100 required!");
} else {
// Call the parent class withdraw method
super.withdraw(amount);
}
}
}
// Main.java
// Main class
// Define the Main class
public class Main {
// Main method, entry point of the program
public static void main(String[] args) {
// Print message to indicate creation of a BankAccount object
System.out.println("Create a Bank Account object (A/c No. BA1234) with initial balance of $500:");
// Create a BankAccount object (A/c No. "BA1234") with initial balance of $500
BankAccount BA1234 = new BankAccount("BA1234", 500);
// Print message to indicate deposit action
System.out.println("Deposit $1000 into account BA1234:");
// Deposit $1000 into account BA1234
BA1234.deposit(1000);
// Print the new balance after deposit
System.out.println("New balance after depositing $1000: $" + BA1234.getBalance());
// Print message to indicate withdrawal action
System.out.println("Withdraw $600 from account BA1234:");
// Withdraw $600 from account BA1234
BA1234.withdraw(600);
// Print the new balance after withdrawal
System.out.println("New balance after withdrawing $600: $" + BA1234.getBalance());
// Print message to indicate creation of a SavingsAccount object
System.out.println("\nCreate a SavingsAccount object (A/c No. SA1234) with initial balance of $450:");
// Create a SavingsAccount object (A/c No. "SA1234") with initial balance of $450
SavingsAccount SA1234 = new SavingsAccount("SA1234", 450);
// Withdraw $300 from SA1234
SA1234.withdraw(300);
// Print the balance after attempting to withdraw $300
System.out.println("Balance after trying to withdraw $300: $" + SA1234.getBalance());
// Print message to indicate creation of another SavingsAccount object
System.out.println("\nCreate a SavingsAccount object (A/c No. SA1000) with initial balance of $300:");
// Create a SavingsAccount object (A/c No. "SA1000") with initial balance of $300
SavingsAccount SA1000 = new SavingsAccount("SA1000", 300);
// Print message to indicate withdrawal action
System.out.println("Try to withdraw $250 from SA1000!");
// Withdraw $250 from SA1000 (balance falls below $100)
SA1000.withdraw(250);
// Print the balance after attempting to withdraw $250
System.out.println("Balance after trying to withdraw $250: $" + SA1000.getBalance());
}
}
Output:
Create a Bank Account object (A/c No. BA1234) with initial balance of $500: Deposit $1000 into account BA1234: New balance after depositing $1000: $1500.0 Withdraw $600 from account BA1234: New balance after withdrawing $600: $900.0 Create a SavingsAccount object (A/c No. SA1234) with initial balance of $450: Balance after trying to withdraw $300: $150.0 Create a SavingsAccount object (A/c No. SA1000) with initial balance of $300: Try to withdraw $250 from SA1000! Minimum balance of $100 required! Balance after trying to withdraw $250: $300.0
Explanation:
The BankAccount class has a constructor that takes account number and balance as arguments. It also has methods to deposit and withdraw money, and to check the account balance.
The SavingsAccount class is a subclass of BankAccount and overrides the withdraw() method. It checks if the account balance falls below one hundred before allowing a withdrawal. The method prints an error message if the balance is below one hundred. If the balance is greater than or equal to one hundred, the method calls the withdraw() method of the superclass to withdraw.
In Main() method -
The main method begins by creating an instance of the BankAccount class with an account number of "BA1234" and an initial balance of $500. It then deposits $1000 into the account and displays the new balance. It then withdraws $600 from the account and displays the new balance.
Next, the method creates an instance of the SavingsAccount class with an account number of "SA1234" and an initial balance of $450. It then attempts to withdraw $300 from the account and displays the new balance. Since the balance remains above the minimum $150 balance required for the account, the withdrawal is successful.
Finally, the method creates another instance of the SavingsAccount class with an account number of "SA1000" and an initial balance of $300. It then attempts to withdraw $250 from the account, which would bring the balance below the minimum balance required for the account. The method displays the new balance after the attempted withdrawal, which should still be $300 since the withdrawal was unsuccessful.
Flowchart:
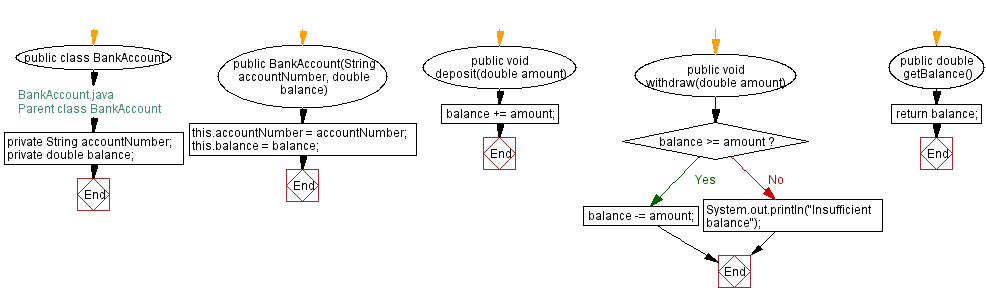
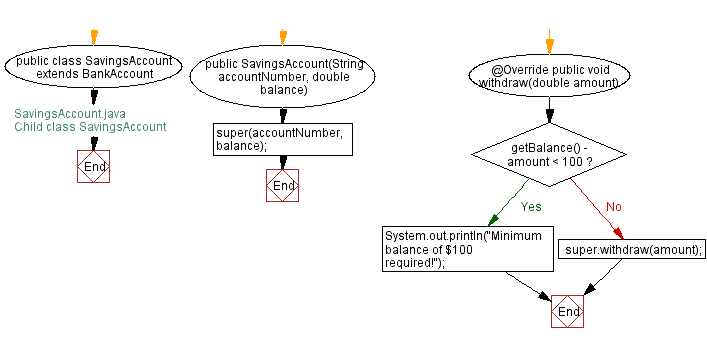
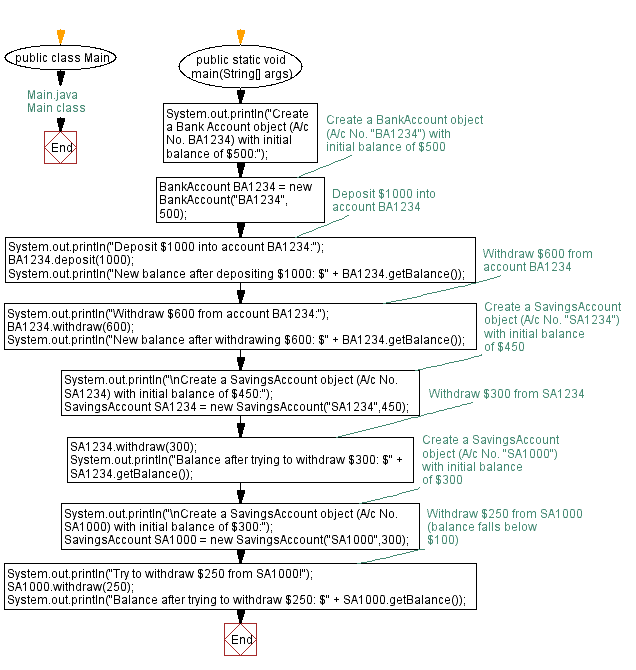
For more Practice: Solve these Related Problems:
- Write a Java program where the "SavingsAccount" subclass automatically transfers excess funds to a fixed deposit.
- Write a Java program where the "BankAccount" class prevents duplicate account numbers.
- Write a Java program where the "SavingsAccount" subclass adds a method to calculate yearly interest.
- Write a Java program where the "BankAccount" class includes fraud detection for unusual transactions.
Go to:
Java Code Editor:
Contribute your code and comments through Disqus.
PREV : Employee class with methods called work, getSalary.
NEXT : Animal Class with a method move() .
What is the difficulty level of this exercise?