Java Inheritance Programming - Animal Class with a method move()
Write a Java program to create a class called Animal with a method named move(). Create a subclass called Cheetah that overrides the move() method to run.
Sample Solution:
Java Code:
// Animal.java
// Parent class Animal
// Define the Animal class
public class Animal {
// Method to print a message indicating the animal moves
public void move() {
System.out.println("Animal moves");
}
}
// Cheetah.java
// Child class Cheetah
// Define the Cheetah class, inheriting from Animal
public class Cheetah extends Animal {
// Override the move method to print a cheetah-specific message
@Override
public void move() {
System.out.println("This cheetah is running!");
}
}
// Main.java
// Main class
// Define the Main class
public class Main {
// Main method to run the program
public static void main(String[] args) {
// Create an instance of Animal
Animal animal = new Animal();
// Call the move method on the Animal instance
animal.move();
// Create an instance of Cheetah
Cheetah cheetah = new Cheetah();
// Call the move method on the Cheetah instance
cheetah.move();
}
}
Output:
Animal moves This cheetah is running!
Explanation:
In the above exercise, the Animal class has a single method called move(). This method simply prints a message to the console saying the animal is moving. The Cheetah class extends the Animal class and overrides the move() method to print a message that Cheetah is running.
In the Main class, we create an instance of the "Animal" class and call its move() method. This prints the "This animal is moving" message to the console. We also create an instance of the "Cheetah" class and call its move() method. This prints the "This cheetah is running" message to the console.
Flowchart:
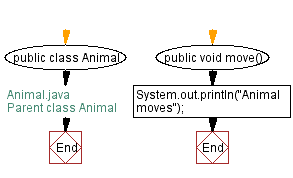
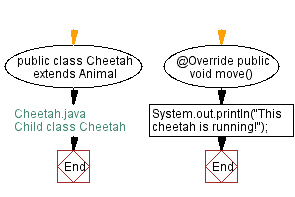
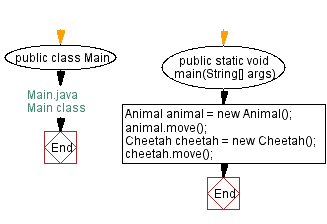
For more Practice: Solve these Related Problems:
- Write a Java program where the "Cheetah" subclass adds a method to track its top running speed.
- Write a Java program where the "Animal" class has a method to track migration patterns.
- Write a Java program where the "Cheetah" subclass includes a method to test reaction speed.
- Write a Java program where the "Animal" class introduces an attribute for diet, and subclasses override it.
Go to:
Java Code Editor:
Contribute your code and comments through Disqus.
PREV : BankAccount class with methods called deposit() and withdraw().
NEXT : Person Class with methods called getFirstName() and getLastName().
What is the difficulty level of this exercise?