Java Polymorphism - Sports Class with Football, Basketball, and Rugby Subclasses for Playing Statements
Java Polymorphism: Exercise-5 with Solution
Write a Java program to create a base class Sports with a method called play(). Create three subclasses: Football, Basketball, and Rugby. Override the play() method in each subclass to play a specific statement for each sport.
In the given exercise, here is a simple diagram illustrating polymorphism implementation:
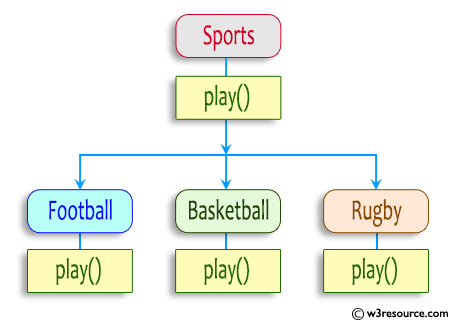
In the above diagram, the Sports class is the base class with a play() method. The Football, Basketball, and Rugby classes are subclasses that inherit from the Sports class and override the play() method to provide their specific implementation.
Sample Solution:
Java Code:
// Sports.java
// Base class Sports
class Sports {
public void play() {
System.out.println("Playing a sport...\n");
}
}
// Football.java
// Subclass Football
class Football extends Sports {
@Override
public void play() {
System.out.println("Playing football...");
}
}
// Basketball.java
// Subclass Basketball
class Basketball extends Sports {
@Override
public void play() {
System.out.println("Playing basketball...");
}
}
// Rugby.java
// Subclass Rugby
class Rugby extends Sports {
@Override
public void play() {
System.out.println("Playing rugby...");
}
}
// Main.java
// Main class
public class Main {
public static void main(String[] args) {
Sports sports = new Sports();
Football football = new Football();
Basketball basketball = new Basketball();
Rugby rugby = new Rugby();
sports.play();
football.play();
basketball.play();
rugby.play();
}
}
Sample Output:
Playing a sport... Playing football... Playing basketball... Playing rugby...
Flowchart:
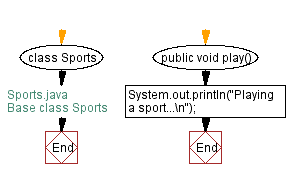
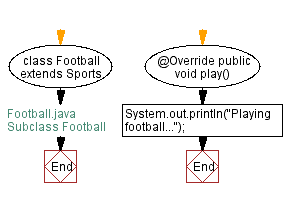
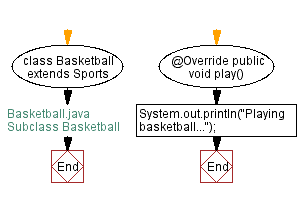
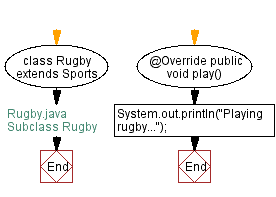
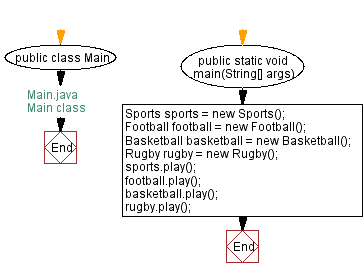
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Shape Class with Circle, Rectangle, and Triangle Subclasses for Area Calculation.
Next: Shape Class with Circle, Rectangle, and Triangle Subclasses for Area and Perimeter Calculation.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics