Java: Calculate the Binomial Coefficient of two positive numbers
Calculate Binomial Coefficient
From Wikipedia,
Write a Java program to calculate the Binomial Coefficient of two positive numbers.
Sample Solution:
Java Code:
import java.util.*;
class solution {
private static long binomial_Coefficient(int n, int k)
{
if (k>n-k)
k=n-k;
long result = 1;
for (int i=1, m=n; i<=k; i++, m--)
result = result*m/i;
return result;
}
public static void main(String[] args)
{
Scanner scan = new Scanner(System.in);
System.out.print("Input the first number(n): ");
int n = scan.nextInt();
System.out.print("Input the second number(k): ");
int k = scan.nextInt();
if (n>0 && k>0)
{
System.out.println("Binomial Coefficient of the said numbers " + binomial_Coefficient(n, k));
}
}
}
Sample Output:
Input the first number(n): 10 Input the second number(k): 2 Binomial Coefficient of the said numbers 45
Flowchart:
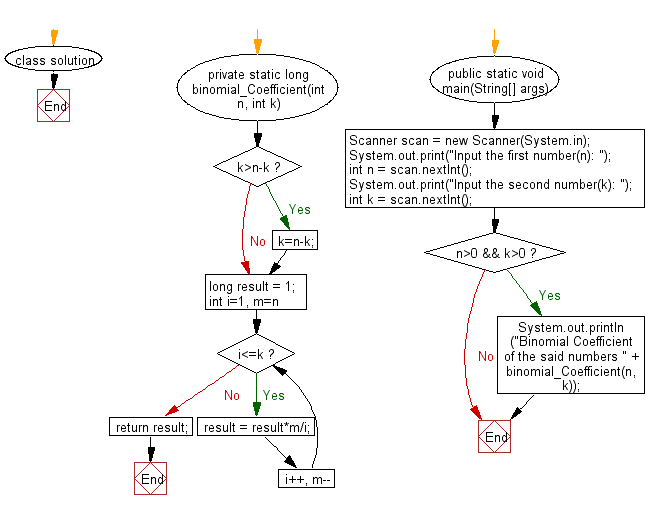
For more Practice: Solve these Related Problems:
- Write a Java program to compute the binomial coefficient using recursion with memoization for efficiency.
- Write a Java program to calculate the binomial coefficient iteratively using Pascal’s Triangle.
- Write a Java program to implement dynamic programming to calculate the binomial coefficient using a two-dimensional array.
- Write a Java program to compute the binomial coefficient recursively and compare the result with an iterative approach.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to compute the result from the innermost brackets.
Next: Write a Java program to calculate e raise to the power x using sum of first n terms of Taylor Series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.