Java: Check whether a number is a Lucky Number or not.
Check Lucky Number
Write a Java program to check whether a number is a Luck number or not.
Lucky numbers are defined via a sieve as follows.
Begin with a list of integers starting with 1 :
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, . . . .
Now eliminate every second number :
1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, ...
The second remaining number is 3, so remove every 3rd number:
1, 3, 7, 9, 13, 15, 19, 21, 25, ...
The next remaining number is 7, so remove every 7th number:
1, 3, 7, 9, 13, 15, 21, 25, ...
Next, remove every 9th number and so on.
Finally, the resulting sequence is the lucky numbers.
Following animation demonstrating the lucky number sieve. The numbers in red are lucky numbers.
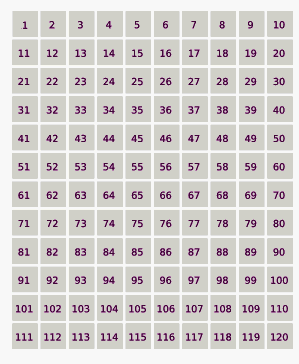
Animation Credits: Celtic Minstrel
Sample Solution:
Java Code:
import java.util.*;
public class solution {
public static boolean isLucky(int n) {
//Create an array of size 10 to initialize all elements as
//false to check if a digit is already seen or not.
boolean temp[]=new boolean[10];
for (int i = 0; i < 10; i++)
temp[i] = false;
while (n > 0)
{
// Find the last digit
int digit = n % 10;
// Return false if digit is already seen,
if (temp[digit])
return false;
temp[digit] = true;
n = n / 10;
}
return true;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Input an integer: ");
String input = scanner.nextLine();
int number = Integer.parseInt(input);
System.out.println("Is Lucky number? "+isLucky(number));
}
}
Sample Output:
Input an integer: 25 Is Lucky number? true
Flowchart:
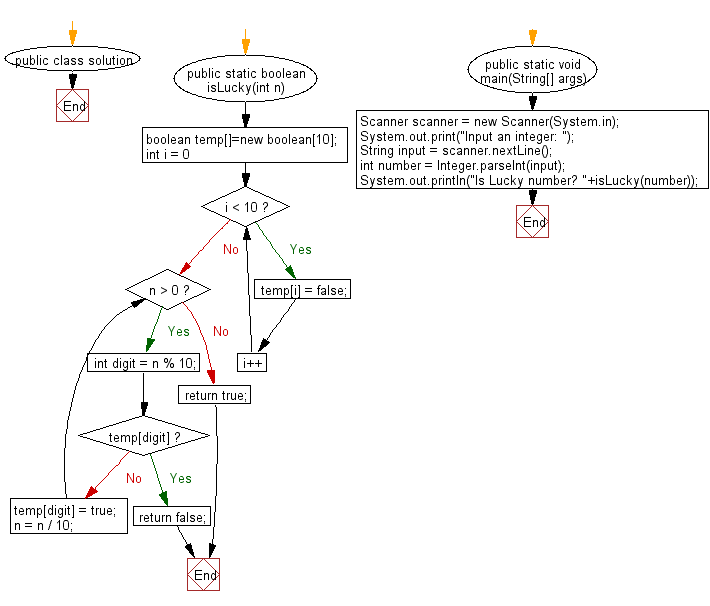
For more Practice: Solve these Related Problems:
- Write a Java program to determine if a number is lucky by recursively eliminating digits in a specified pattern.
- Write a Java program to generate lucky numbers within a given range using iterative filtering.
- Write a Java program to compare the sum of digits at odd and even positions to decide if a number is lucky.
- Write a Java program to implement a lucky number checker using a custom algorithm based on digit patterns.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Program in Java to check whether a number is an Armstrong Number or not.
Next: Java Input-Output Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.