Java: Restaurant menu, average rating
Java OOP: Exercise-18 with Solution
Write a Java program to create a class called "Restaurant" with attributes for menu items, prices, and ratings, and methods to add and remove items, and to calculate average rating.
Sample Solution:
Java Code:
// Restaurant.java
// Import the ArrayList class
import java.util.ArrayList;
// Define the Restaurant class
public class Restaurant {
// Declare ArrayLists to store menu items, prices, ratings, and item counts
private ArrayList menuItems;
private ArrayList prices;
private ArrayList ratings;
private ArrayList itemCounts;
// Constructor to initialize the ArrayLists
public Restaurant() {
// Initialize the menuItems ArrayList
this.menuItems = new ArrayList();
// Initialize the prices ArrayList
this.prices = new ArrayList();
// Initialize the ratings ArrayList
this.ratings = new ArrayList();
// Initialize the itemCounts ArrayList
this.itemCounts = new ArrayList();
}
// Method to add an item to the menu
public void addItem(String item, double price) {
// Add the item to the menuItems ArrayList
this.menuItems.add(item);
// Add the price to the prices ArrayList
this.prices.add(price);
// Initialize the rating for the item to 0
this.ratings.add(0);
// Initialize the item count for the item to 0
this.itemCounts.add(0);
}
// Method to remove an item from the menu
public void removeItem(String item) {
// Get the index of the item in the menuItems ArrayList
int index = this.menuItems.indexOf(item);
// If the item exists in the menu
if (index >= 0) {
// Remove the item from the menuItems ArrayList
this.menuItems.remove(index);
// Remove the corresponding price from the prices ArrayList
this.prices.remove(index);
// Remove the corresponding rating from the ratings ArrayList
this.ratings.remove(index);
// Remove the corresponding item count from the itemCounts ArrayList
this.itemCounts.remove(index);
}
}
// Method to add a rating to an item
public void addRating(String item, int rating) {
// Get the index of the item in the menuItems ArrayList
int index = this.menuItems.indexOf(item);
// If the item exists in the menu
if (index >= 0) {
// Get the current rating of the item
int currentRating = this.ratings.get(index);
// Get the current item count of the item
int totalCount = this.itemCounts.get(index);
// Update the rating of the item
this.ratings.set(index, currentRating + rating);
// Update the item count of the item
this.itemCounts.set(index, totalCount + 1);
}
}
// Method to get the average rating of an item
public double getAverageRating(String item) {
// Get the index of the item in the menuItems ArrayList
int index = this.menuItems.indexOf(item);
// If the item exists in the menu
if (index >= 0) {
// Get the total rating of the item
int totalRating = this.ratings.get(index);
// Get the item count of the item
int itemCount = this.itemCounts.get(index);
// Calculate and return the average rating of the item
return itemCount > 0 ? (double) totalRating / itemCount : 0.0;
} else {
// Return 0.0 if the item does not exist in the menu
return 0.0;
}
}
// Method to display the menu
public void displayMenu() {
// Loop through the menuItems ArrayList
for (int i = 0; i < menuItems.size(); i++) {
// Print the item and its price
System.out.println(menuItems.get(i) + ": $" + prices.get(i));
}
}
// Method to calculate the average rating of all items
public double calculateAverageRating() {
// Initialize totalRating to 0
double totalRating = 0;
// Initialize numRatings to 0
int numRatings = 0;
// Loop through the ratings ArrayList
for (int i = 0; i < ratings.size(); i++) {
// Add the rating to totalRating
totalRating += ratings.get(i);
// Increment numRatings
numRatings++;
}
// Calculate and return the average rating
return numRatings > 0 ? totalRating / numRatings : 0.0;
}
}
The above Java class defines a restaurant with menu items, prices, and ratings. It has a constructor that initializes three ArrayLists for the menu items, prices, and ratings. It also has methods to add and remove items from the menu and add ratings for each item. The class also includes a method to calculate the average rating for a given menu item. It also includes a method to display the current menu.
// Main.java
// Define the Main class
public class Main {
// Main method, the entry point of the application
public static void main(String[] args) {
// Create a new Restaurant object
Restaurant restaurant = new Restaurant();
// Add a Burger item with a price of $8.99 to the menu
restaurant.addItem("Burger", 8.99);
// Add a Pizza item with a price of $10.99 to the menu
restaurant.addItem("Pizza", 10.99);
// Add a Salad item with a price of $6.00 to the menu
restaurant.addItem("Salad", 6.00);
// Print the menu header
System.out.println("Menu: Item & Price");
// Display the menu items and their prices
restaurant.displayMenu();
// Add a rating of 4 to the Burger item
restaurant.addRating("Burger", 4);
// Add a rating of 5 to the Burger item
restaurant.addRating("Burger", 5);
// Add a rating of 3 to the Pizza item
restaurant.addRating("Pizza", 3);
// Add a rating of 4 to the Pizza item
restaurant.addRating("Pizza", 4);
// Add a rating of 2 to the Salad item
restaurant.addRating("Salad", 2);
// Get the average rating for the Burger item
double averageRating = restaurant.getAverageRating("Burger");
// Print the average rating for the Burger item
System.out.println("\nAverage rating for Burger: " + averageRating);
// Get the average rating for the Pizza item
averageRating = restaurant.getAverageRating("Pizza");
// Print the average rating for the Pizza item
System.out.println("Average rating for Pizza: " + averageRating);
// Get the average rating for the Salad item
averageRating = restaurant.getAverageRating("Salad");
// Print the average rating for the Salad item
System.out.println("Average rating for Salad: " + averageRating);
// Print the overall average rating for all items
System.out.println("Average rating: " + restaurant.calculateAverageRating());
// Print a message indicating that the Pizza item will be removed
System.out.println("\nRemove 'Pizza' from the above menu.");
// Remove the Pizza item from the menu
restaurant.removeItem("Pizza");
// Print the updated menu header
System.out.println("\nUpdated menu:");
// Display the updated menu items and their prices
restaurant.displayMenu();
}
}
The Main class contains the main function that creates an object of the Restaurant class and calls its methods to add, remove, and display menu items, as well as add ratings and calculate average ratings for those items.
Sample Output:
Menu: Burger: $8.99 Pizza: $10.99 Salad: $6.0 Average rating: 4.666666666666667 Remove 'Pizza' from the above menu. Updated menu: Burger: $8.99 Salad: $6.0
Flowchart:
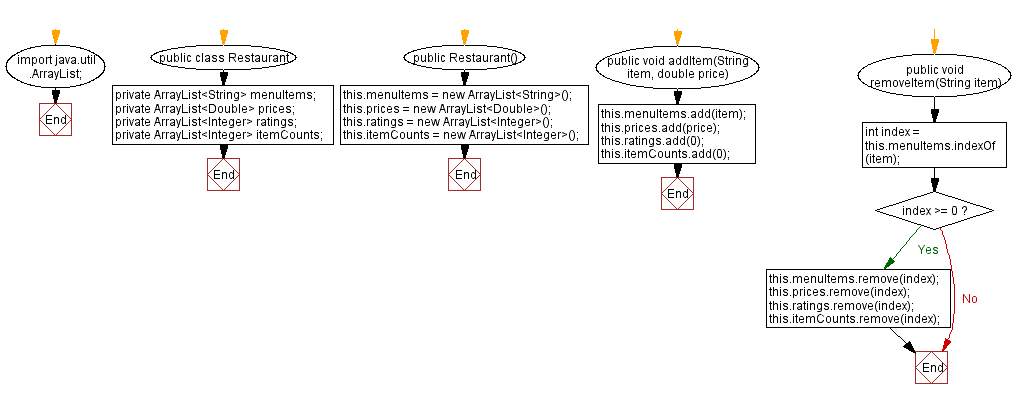
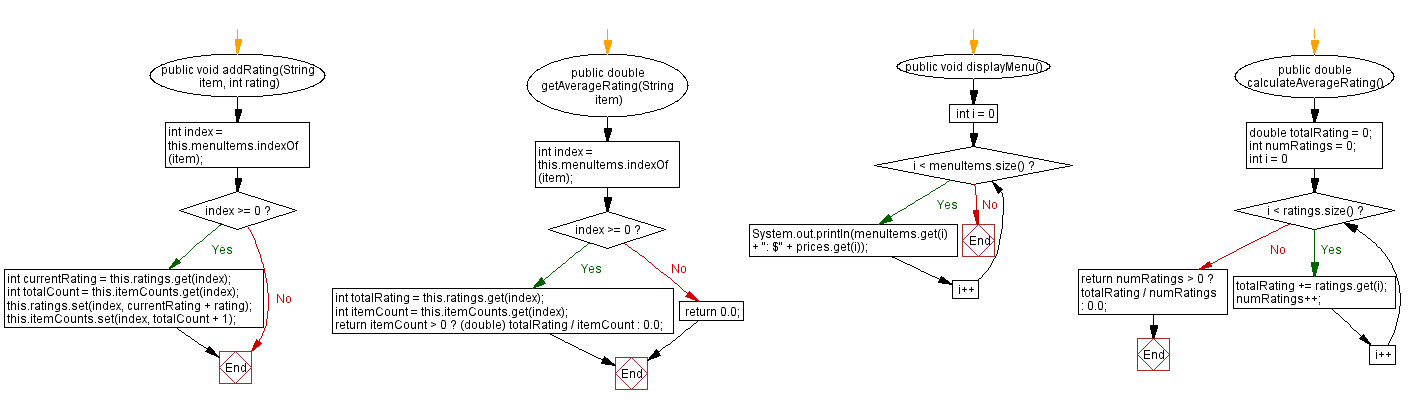
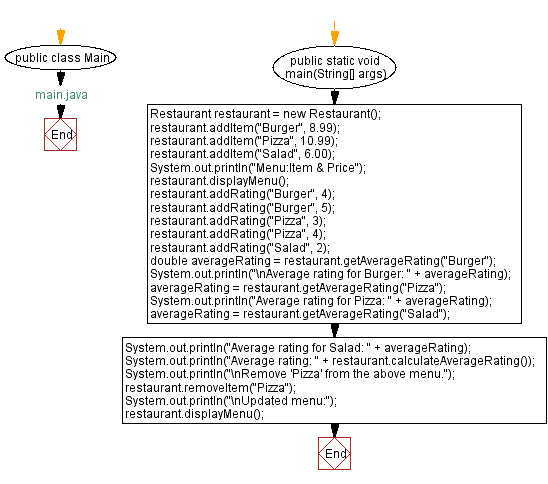
Java Code Editor:
Improve this sample solution and post your code through Disqus.
Java OOP Previous: Movie and Review.
Java OOP Next: Search, book, cancel hotel and flight reservations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/oop/java-oop-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics