Java: CountingSort Algorithm
12. Counting Sort Implementation
Write a Java program to sort an array of given integers using the CountingSort algorithm.
According to Wikipedia "In computer science, counting sort is an algorithm for sorting a collection of objects according to keys that are small integers; that is, it is an integer sorting algorithm. It operates by counting the number of objects that have each distinct key value. It uses arithmetic on those counts to determine the positions of each key value in the output sequence. Its running time is linear based on the number of items and the difference between the maximum and minimum key values, so it is only suitable for direct use in situations where the variation in keys is not significantly wider than the number of items. However, it is often used as a subroutine in another sorting algorithm, radix sort, which handles larger keys more efficiently".
The algorithm loops over the items, computing a histogram of the number of times each key occurs within the input collection. It then performs a prefix sum computation (a second loop, over the range of possible keys) to determine, for each key, the starting position in the output array of the items having that key. Finally, it loops over the items again, moving each item into its sorted position in the output array.
Sample Solution:
Java Code:
import java.util.Arrays;
class countingSort {
void countingSort(int[] nums, int min, int max){
int[] ctr = new int[max - min + 1];
for(int number : nums){
ctr[number - min]++;
}
int z= 0;
for(int i= min;i <= max;i++){
while(ctr[i - min] > 0){
nums[z]= i;
z++;
ctr[i - min]--;
}
}
}
// Method to test above
public static void main(String args[])
{
countingSort ob = new countingSort();
int nums[] = {7, -5, 3, 2, 1, 0, 45};
int minValue = -5, maxValue = 45;
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.countingSort(nums,minValue,maxValue);
System.out.println("Sorted Array");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
Original Array: [7, -5, 3, 2, 1, 0, 45] Sorted Array [-5, 0, 1, 2, 3, 7, 45]
Flowchart:
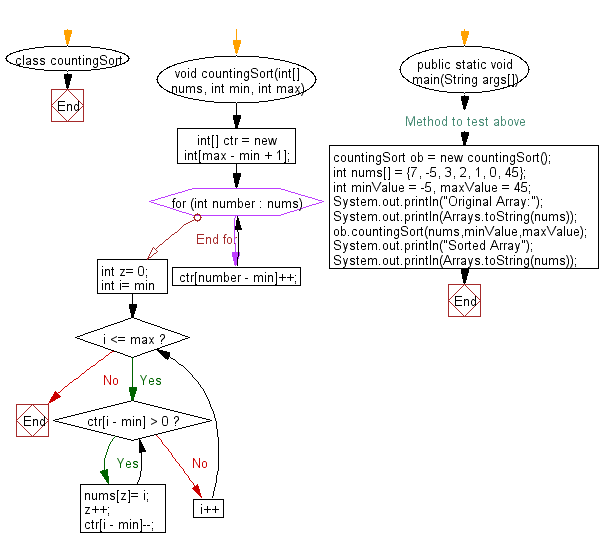
For more Practice: Solve these Related Problems:
- Write a Java program to implement counting sort for an array of integers and handle negative numbers.
- Write a Java program to modify counting sort to sort an array of characters.
- Write a Java program to implement counting sort and optimize memory usage when the range of keys is large.
- Write a Java program to create a stable counting sort that preserves the original order of equal elements.
Go to:
PREV : Comb Sort Implementation.
NEXT : Gnome Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.