Java: CombSort Algorithm
Write a Java program to sort an array of given integers using the CombSort algorithm.
The Comb Sort is a variant of the Bubble Sort. Like the Shell sort, the Comb Sort increases the gap used in comparisons and exchanges. Some implementations use the insertion sort once the gap is less than a certain amount. The basic idea is to eliminate turtles, or small values near the end of the list, since in a bubble sort these slow the sorting down tremendously. Rabbits, large values around the beginning of the list do not pose a problem in a bubble sort.
In bubble sort, when any two elements are compared, they always have a gap of 1. The basic idea of comb sort is that the gap can be much more than 1.
Visualization of comb sort :
Animation credits : Jerejesse
Sample Solution:
Java Code:
import java.util.Arrays;
class CombSort {
void CombSort(int nums[]){
int gap_length = nums.length;
float shrinkFactor = 1.3f;
boolean swapped = false;
while (gap_length > 1 || swapped) {
if (gap_length > 1) {
gap_length = (int)(gap_length / shrinkFactor);
}
swapped = false;
for (int i = 0; gap_length + i < nums.length; i++) {
if (nums[i] > nums[i + gap_length]) {
swap(nums, i, i + gap_length);
swapped = true;
}
}
}
}
private static void swap(int nums[], int x, int y) {
Integer temp = nums[x];
nums[x] = nums[y];
nums[y] = temp;
}
// Method to test above
public static void main(String args[])
{
CombSort ob = new CombSort();
int nums[] = {7, -5, 3, 2, 1, 0, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.CombSort(nums);
System.out.println("Sorted Array");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
Original Array: [7, -5, 3, 2, 1, 0, 45] Sorted Array [-5, 0, 1, 2, 3, 7, 45]
Flowchart:
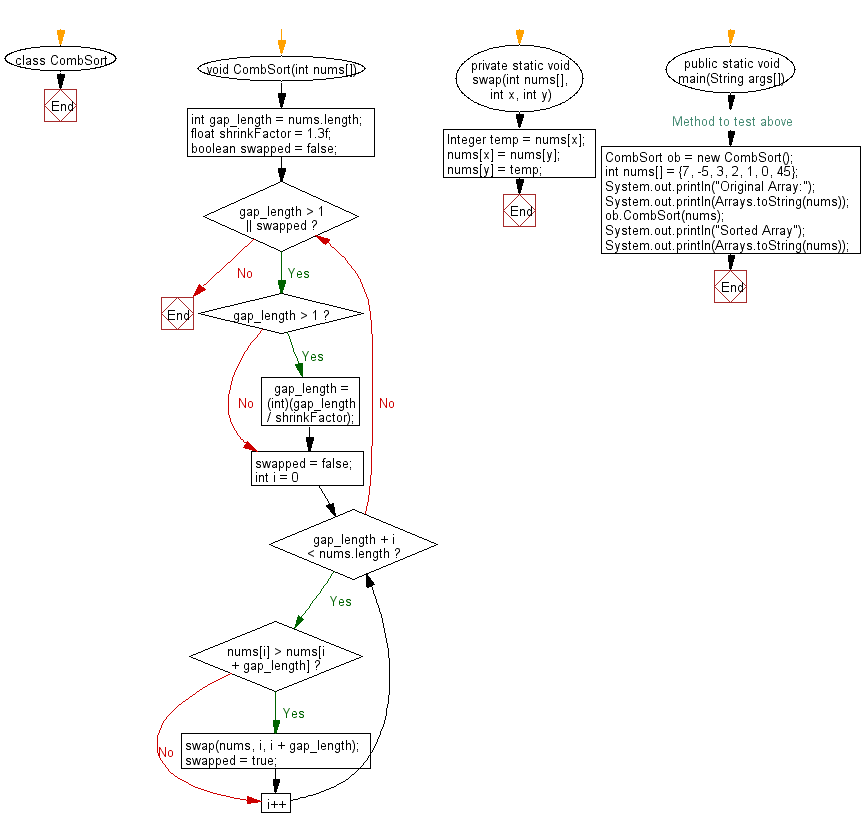
For more Practice: Solve these Related Problems:
- Write a Java program to implement comb sort and experiment with different shrink factors for optimization.
- Write a Java program to extend comb sort to sort an array of objects using a custom comparator.
- Write a Java program to implement comb sort and analyze its performance on nearly sorted data.
- Write a Java program to modify comb sort to switch to bubble sort when the gap becomes very small.
Go to:
PREV : Cocktail Sort Implementation.
NEXT : Counting Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.