Java: Cocktail sort Algorithm
Java Sorting Algorithm: Exercise-10 with Solution
Write a Java program to sort an array of positive integers using the Cocktail sort algorithm.
Cocktail shaker sort (also known as bidirectional bubble sort, cocktail sort, shaker sort, ripple sort, shuffle sort, or shuttle sort ) is a variation of bubble sort that is both a stable sorting algorithm and a comparison sort. The algorithm differs from a bubble sort in that it sorts in both directions on each pass through the list. This sorting algorithm is only marginally more difficult to implement than a bubble sort and solves the problem of turtles in bubble sorts. It provides only marginal performance improvements, and does not improve asymptotic performance; like the bubble sort, it is not of practical interest, though it finds some use in education.
Visualization of shaker sort :
Sample Solution:
Java Code:
import java.util.Arrays;
class cocktailSort
{
void cocktailSort(int nums[])
{
boolean swapped;
do {
swapped = false;
for (int i =0; i<= nums.length - 2;i++) {
if (nums[ i ] > nums[ i + 1 ]) {
//test if two elements are in the wrong order
int temp = nums[i];
nums[i] = nums[i+1];
nums[i+1]=temp;
swapped = true;
}
}
if (!swapped) {
break;
}
swapped = false;
for (int i= nums.length - 2;i>=0;i--) {
if (nums[ i ] > nums[ i + 1 ]) {
int temp = nums[i];
nums[i] = nums[i+1];
nums[i+1]=temp;
swapped = true;
}
}
} while (swapped);
}
// Method to test above
public static void main(String args[])
{
cocktailSort ob = new cocktailSort();
int nums[] = {7, 5, 3, 2, 1, 12, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.cocktailSort(nums);
System.out.println("Sorted Array");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
Original Array: [7, 5, 3, 2, 1, 12, 45] Sorted Array [1, 2, 3, 5, 7, 12, 45]
Flowchart:
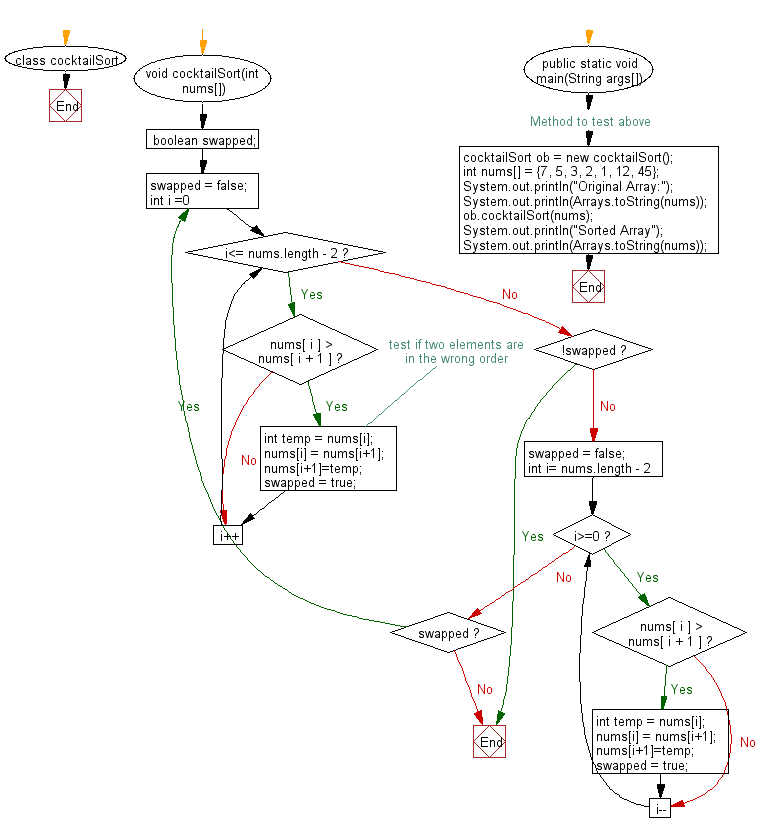
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to sort an array of positive integers using the BogoSort Sort Algorithm.
Next: Write a Java program to sort an array of given integers using the CombSort Algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/sorting/java-sorting-algorithm-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics