Java: BogoSort Sort Algorithm
Java Sorting Algorithm: Exercise-9 with Solution
Write a Java program to sort an array of positive integers using the BogoSort Sort Algorithm.
In computer science, Bogosort is a particularly ineffective sorting algorithm based on the generate and test paradigm. The algorithm successively generates permutations of its input until it finds one that is sorted. It is not useful for sorting but may be used for educational purposes, to contrast it with other more realistic algorithms.
Sample Solution:
Java Code:
public class BogoSort
{
public static void main(String[] args)
{
//Enter array to be sorted here
int[] arr={4,5,6,0,7,8,9,1,2,3};
BogoSort now=new BogoSort();
System.out.print("Unsorted: ");
now.display1D(arr);
now.bogo(arr);
System.out.print("Sorted: ");
now.display1D(arr);
}
void bogo(int[] arr)
{
//Keep a track of the number of shuffles
int shuffle=1;
for(;!isSorted(arr);shuffle++)
shuffle(arr);
//Boast
System.out.println("This took "+shuffle+" shuffles.");
}
void shuffle(int[] arr)
{
//Standard Fisher-Yates shuffle algorithm
int i=arr.length-1;
while(i>0)
swap(arr,i--,(int)(Math.random()*i));
}
void swap(int[] arr,int i,int j)
{
int temp=arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
boolean isSorted(int[] arr)
{
for(int i=1;i<arr.length;i++)
if(arr[i]<arr[i-1])
return false;
return true;
}
void display1D(int[] arr)
{
for(int i=0;i<arr.length;i++)
System.out.print(arr[i]+" ");
System.out.println();
}
}
Sample Output:
Unsorted: 4 5 6 0 7 8 9 1 2 3 This took 3067022 shuffles. Sorted: 0 1 2 3 4 5 6 7 8 9
Flowchart:
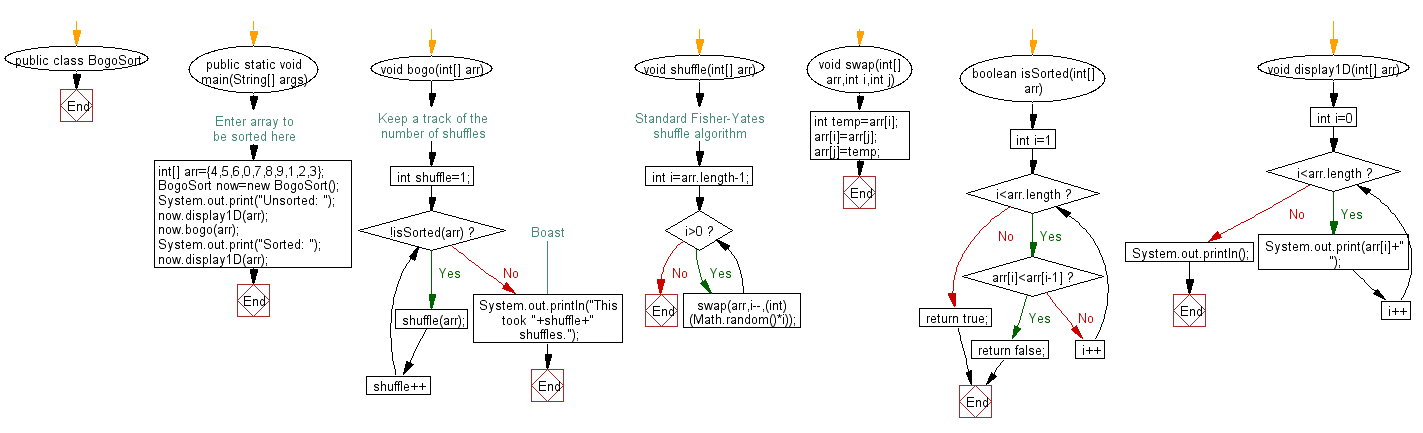
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to sort an array of positive integers using the Bead Sort Algorithm.
Next: Write a Java program to sort an array of given integers using the Cocktail sort Algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/sorting/java-sorting-algorithm-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics