Java: Bead Sort Algorithm
8. Bead Sort Implementation
Write a Java program to sort an array of positive integers using the Bead Sort Algorithm.
According to Wikipedia "Bead sort, also called gravity sort, is a natural sorting algorithm, developed by Joshua J. Arulanandham, Cristian S. Calude and Michael J. Dinneen in 2002, and published in The Bulletin of the European Association for Theoretical Computer Science. Both digital and analog hardware implementations of bead sort can achieve a sorting time of O(n); however, the implementation of this algorithm tends to be significantly slower in software and can only be used to sort lists of positive integers. Also, it seems that even in the best case, the algorithm requires O(n2) space".
Sample Solution:
Java Code:
public class BeadSort
{
public static void main(String[] args)
{
BeadSort now=new BeadSort();
int[] arr=new int[(int)(Math.random()*11)+5];
for(int i=0;i<arr.length;i++)
arr[i]=(int)(Math.random()*10);
System.out.print("Unsorted: ");
now.display1D(arr);
int[] sort=now.beadSort(arr);
System.out.print("Sorted: ");
now.display1D(sort);
}
int[] beadSort(int[] arr)
{
int max=0;
for(int i=0;i<arr.length;i++)
if(arr[i]>max)
max=arr[i];
//Set up abacus
char[][] grid=new char[arr.length][max];
int[] levelcount=new int[max];
for(int i=0;i<max;i++)
{
levelcount[i]=0;
for(int j=0;j<arr.length;j++)
grid[j][i]='_';
}
/*
display1D(arr);
display1D(levelcount);
display2D(grid);
*/
//Drop the beads
for(int i=0;i<arr.length;i++)
{
int num=arr[i];
for(int j=0;num>0;j++)
{
grid[levelcount[j]++][j]='*';
num--;
}
}
System.out.println();
display2D(grid);
//Count the beads
int[] sorted=new int[arr.length];
for(int i=0;i<arr.length;i++)
{
int putt=0;
for(int j=0;j<max&&grid[arr.length-1-i][j]=='*';j++)
putt++;
sorted[i]=putt;
}
return sorted;
}
void display1D(int[] arr)
{
for(int i=0;i<arr.length;i++)
System.out.print(arr[i]+" ");
System.out.println();
}
void display1D(char[] arr)
{
for(int i=0;i<arr.length;i++)
System.out.print(arr[i]+" ");
System.out.println();
}
void display2D(char[][] arr)
{
for(int i=0;i<arr.length;i++)
display1D(arr[i]);
System.out.println();
}
}
Sample Output:
Unsorted: 8 2 2 3 4 6 7 8 4 0 8 * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * _ * * * * * * _ _ * * * * _ _ _ _ * * * * _ _ _ _ * * * _ _ _ _ _ * * _ _ _ _ _ _ * * _ _ _ _ _ _ _ _ _ _ _ _ _ _ Sorted: 0 2 2 3 4 4 6 7 8 8 8
Flowchart:
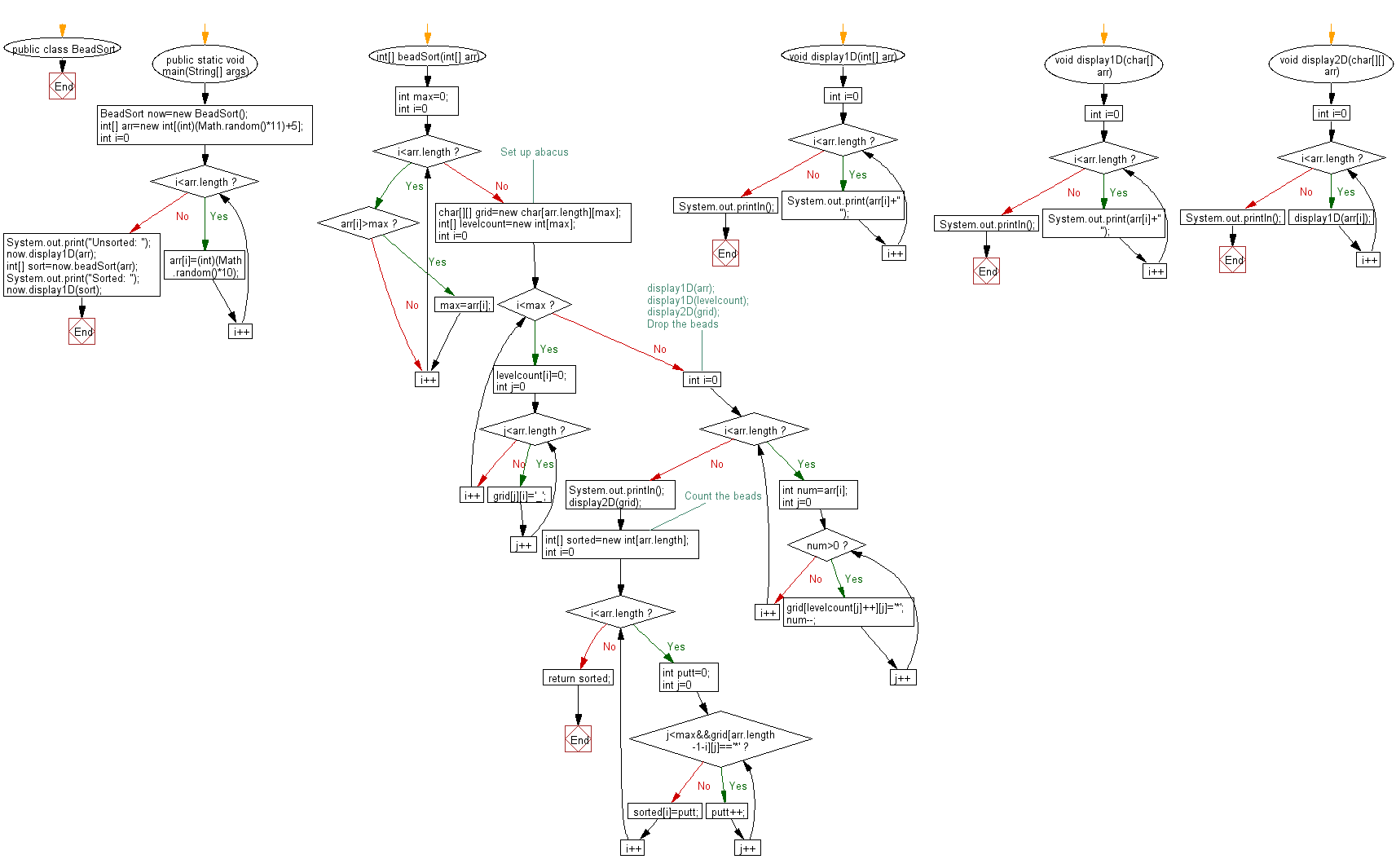
For more Practice: Solve these Related Problems:
- Write a Java program to implement bead sort for an array of positive integers and demonstrate its behavior on nearly sorted data.
- Write a Java program to optimize bead sort by simulating the gravity effect using multi-threading.
- Write a Java program to implement bead sort and compare its performance with bubble sort on small datasets.
- Write a Java program to extend bead sort to visually display the sorting process using console graphics.
Go to:
PREV : Insertion Sort Implementation.
NEXT : Bogo Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.