Java: Insertion sort Algorithm
7. Insertion Sort Implementation
Write a Java program to sort an array of given integers using the Insertion sort algorithm.
Note:
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. It is much less efficient on large lists than other algorithms such as quicksort, heapsort, or merge sort.
Pictorial presentation - Insertion search algorithm :
Sample Solution:
Java Code:
import java.util.Arrays;
public class InsertionSort {
void InsertionSort(int[] nums){
for(int i = 1; i < nums.length; i++){
int value = nums[i];
int j = i - 1;
while(j >= 0 && nums[j] > value){
nums[j + 1] = nums[j];
j = j - 1;
}
nums[j + 1] = value;
}
}
// Method to test above
public static void main(String args[])
{
InsertionSort ob = new InsertionSort();
int nums[] = {7, -5, 3, 2, 1, 0, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.InsertionSort(nums);
System.out.println("Sorted Array");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
[7, -5, 3, 2, 1, 0, 45] Sorted Array [-5, 0, 1, 2, 3, 7, 45]
Flowchart:
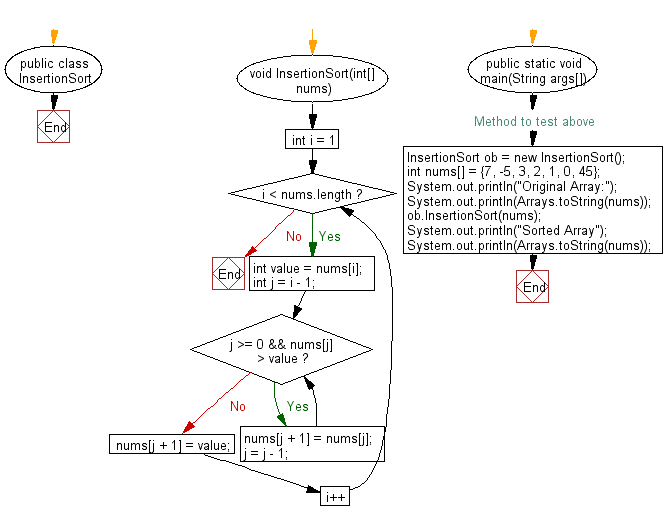
For more Practice: Solve these Related Problems:
- Write a Java program to implement insertion sort and optimize it using binary search for the insertion point.
- Write a Java program to implement insertion sort on a linked list instead of an array.
- Write a Java program to modify insertion sort to sort a partially sorted array efficiently.
- Write a Java program to implement insertion sort and count the number of shifts performed during sorting.
Go to:
PREV : Selection Sort Implementation.
NEXT : Bead Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.