Java: Selection Sort Algorithm
6. Selection Sort Implementation
Write a Java program to sort an array of given integers using the Selection Sort Algorithm.
Wikipedia says "In computer science, selection sort is a sorting algorithm, specifically an in-place comparison sort. It has O(n2) time complexity, making it inefficient on large lists, and generally performs less efficiently than the similar insertion sort".
Note:
a) To find maximum of elements
b) To swap two elements
Pictorial presentation - Selection search algorithm :
Sample Solution:
Java Code:
import java.util.Arrays;
public class SelectionSort {
public static void sort(int[] nums)
{
for(int currentPlace = 0;currentPlace<nums.length-1;currentPlace++){
int smallest = Integer.MAX_VALUE;
int smallestAt = currentPlace+1;
for(int check = currentPlace; check<nums.length;check++){
if(nums[check]<smallest){
smallestAt = check;
smallest = nums[check];
}
}
int temp = nums[currentPlace];
nums[currentPlace] = nums[smallestAt];
nums[smallestAt] = temp;
}
}
// Method to test above
public static void main(String args[])
{
SelectionSort ob = new SelectionSort();
int nums[] = {7, -5, 3, 2, 1, 0, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.sort(nums);
System.out.println("Sorted Array:");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
Original Array: [7, -5, 3, 2, 1, 0, 45] Sorted Array: [-5, 0, 1, 2, 3, 7, 45]
Flowchart:
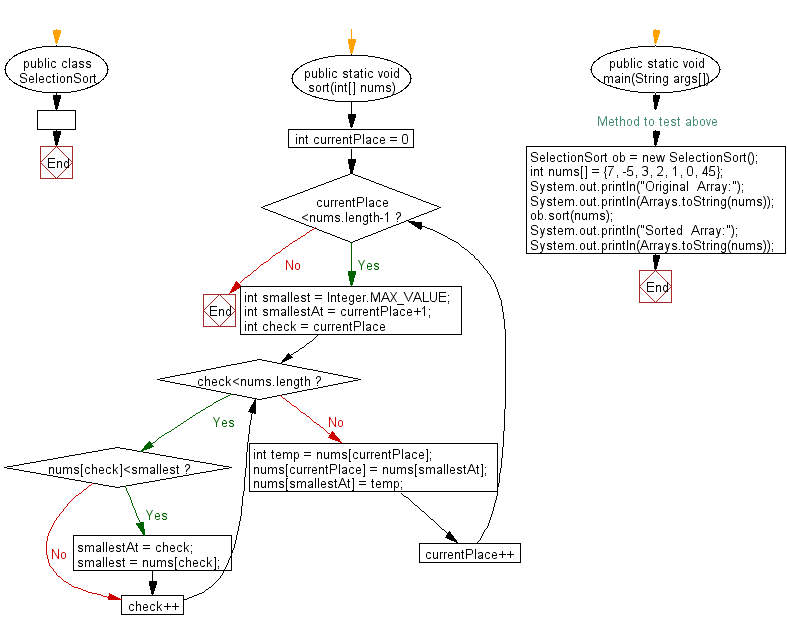
For more Practice: Solve these Related Problems:
- Write a Java program to implement selection sort and simultaneously track the index of the minimum element.
- Write a Java program to modify selection sort to sort an array of objects using a custom comparator.
- Write a Java program to implement selection sort recursively without using loops.
- Write a Java program to implement selection sort and count the number of comparisons made during the process.
Go to:
PREV : Heap Sort Implementation.
NEXT : Insertion Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.