Java: Shell Sort Algorithm
16. Shell Sort Implementation
Write a Java program to sort an array of given integers Shell Sort Algorithm.
According to Wikipedia "Shell sort or Shell's method, is an in-place comparison sort. It can be seen as either a generalization of sorting by exchange (bubble sort) or sorting by insertion (insertion sort). The method starts by sorting pairs of elements far apart from each other, then progressively reducing the gap between elements to be compared. Starting with far apart elements can move some out-of-place elements into position faster than a simple nearest neighbor exchange."
Sample Solution:
Java Code:
import java.util.Arrays;
public class ShellSort {
public static void shell(int[] a) {
int increment = a.length / 2;
while (increment > 0) {
for (int i = increment; i < a.length; i++) {
int j = i;
int temp = a[i];
while (j >= increment && a[j - increment] > temp) {
a[j] = a[j - increment];
j = j - increment;
}
a[j] = temp;
}
if (increment == 2) {
increment = 1;
} else {
increment *= (5.0 / 11);
}
}
}
// Method to test above
public static void main(String args[])
{
ShellSort ob = new ShellSort();
int nums[] = {7, -5, 3, 2, 1, 0, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.shell(nums);
System.out.println("Sorted Array:");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
Original Array: [7, -5, 3, 2, 1, 0, 45] Sorted Array: [-5, 0, 1, 2, 3, 7, 45]
Flowchart:
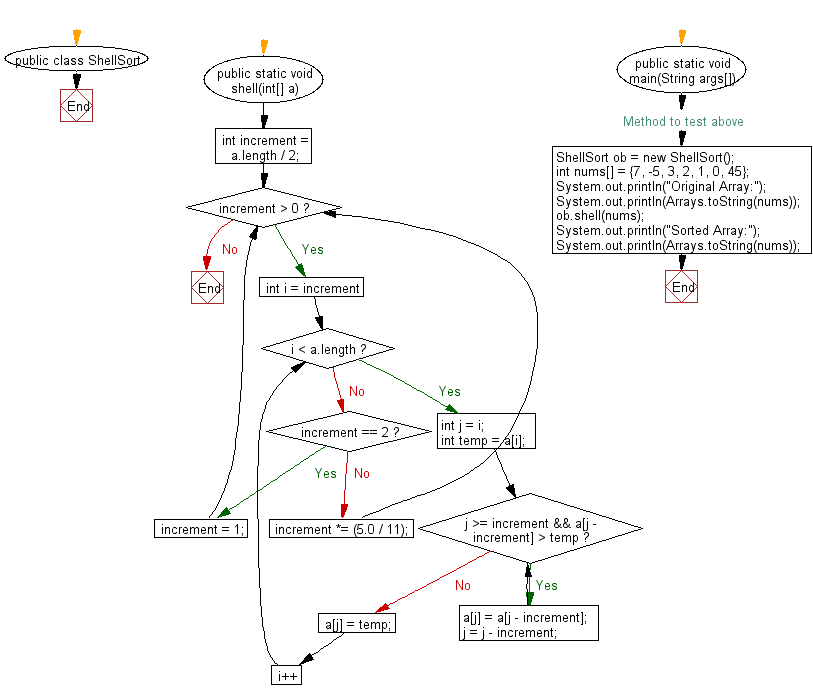
For more Practice: Solve these Related Problems:
- Write a Java program to implement shell sort and experiment with different gap sequences for performance comparison.
- Write a Java program to extend shell sort to work on an array of custom objects using a provided comparator.
- Write a Java program to implement shell sort and measure the number of comparisons and exchanges made.
- Write a Java program to modify shell sort to switch to insertion sort when the gap reduces to one.
Go to:
PREV : Permutation Sort Implementation.
NEXT : Sleep Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.