Java: Sleep Sort Algorithm
17. Sleep Sort Implementation
Write a Java program to sort an array of given non-negative integers using the Sleep Sort Algorithm.
Sleep sort works by starting a separate task for each item to be sorted. Each task sleeps for an interval corresponding to the item's sort key, then emits the item. Items are then collected sequentially in time.
Sample Solution:
Java Code:
import java.util.concurrent.CountDownLatch;
public class SleepSort {
public static void sleepSortAndPrint(int[] nums) {
final CountDownLatch doneSignal = new CountDownLatch(nums.length);
for (final int num : nums) {
new Thread(new Runnable() {
public void run() {
doneSignal.countDown();
try {
doneSignal.await();
//using straight milliseconds produces unpredictable
//results with small numbers
//using 1000 here gives a nifty demonstration
Thread.sleep(num * 500);
System.out.println(num);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
}
}
public static void main(String[] args) {
int[] nums ={7, 3, 2, 1, 0, 5};
for (int i = 0; i < args.length; i++)
nums[i] = Integer.parseInt(args[i]);
sleepSortAndPrint(nums);
}
}
Sample Output:
0 1 2 3 5 7
Flowchart:
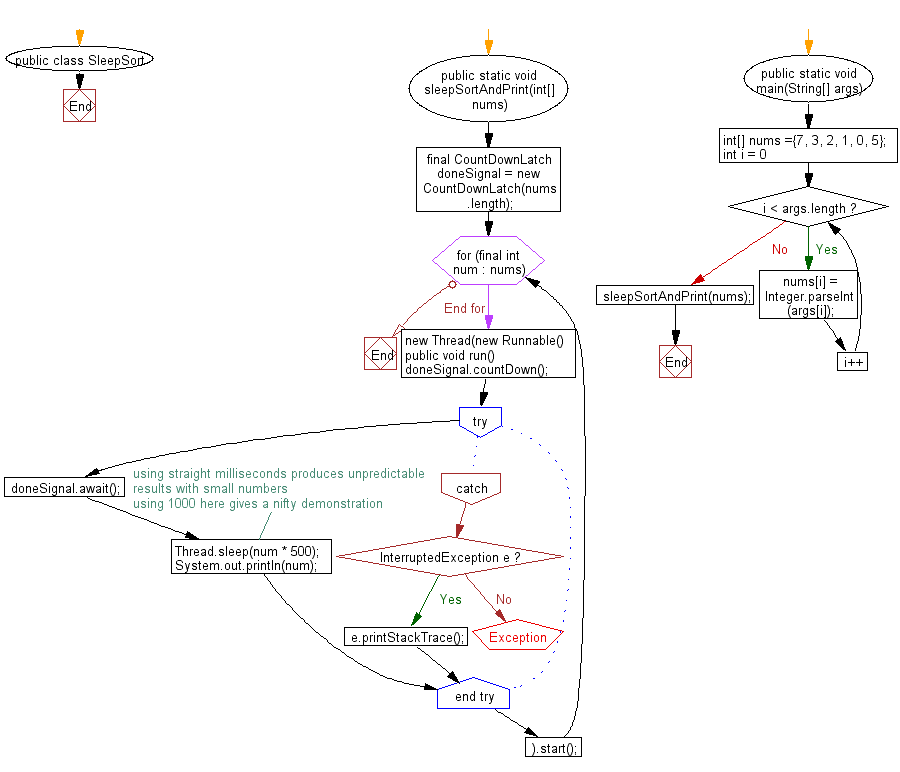
For more Practice: Solve these Related Problems:
- Write a Java program to implement sleep sort using multi-threading to sort an array of non-negative integers.
- Write a Java program to modify sleep sort to handle arrays with duplicate values and output sorted results.
- Write a Java program to implement sleep sort and compare its runtime with other simple sorting algorithms on small arrays.
- Write a Java program to simulate sleep sort by printing each number as it is "awakened" after its corresponding sleep duration.
Go to:
PREV : Shell Sort Implementation.
NEXT : Stooge Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.