Java Exercises: Bubble sort Algorithm
2. Bubble Sort Implementation
Write a Java program to sort an array of given integers using the Bubble Sorting Algorithm.
According to Wikipedia "Bubble sort, sometimes called sinking sort, is a simple sorting algorithm that repeatedly steps through the list to be sorted. It compares each pair of adjacent items and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates the list is in order. The algorithm, which is a comparison sort, is named for the way smaller elements "bubble" to the top of the list. Although the algorithm is simple, it is too slow and impractical for most problems even compared to an insertion sort. It can be practical if the input is usually in sort order but occasionally has some out-of-order elements nearly in position."
Sample Solution:
Java Code:
import java.util.Arrays;
class BubbleSort
{
void bubbleSort(int nums[])
{
int n = nums.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (nums[j] > nums[j+1])
{
// swap temp and nums[i]
int temp = nums[j];
nums[j] = nums[j+1];
nums[j+1] = temp;
}
}
// Method to test above
public static void main(String args[])
{
BubbleSort ob = new BubbleSort();
int nums[] = {7, -5, 3, 2, 1, 0, 45};
System.out.println("Original Array:");
System.out.println(Arrays.toString(nums));
ob.bubbleSort(nums);
System.out.println("Sorted Array");
System.out.println(Arrays.toString(nums));
}
}
Sample Output:
Original Array: [7, -5, 3, 2, 1, 0, 45] Sorted Array [-5, 0, 1, 2, 3, 7, 45]
Flowchart:
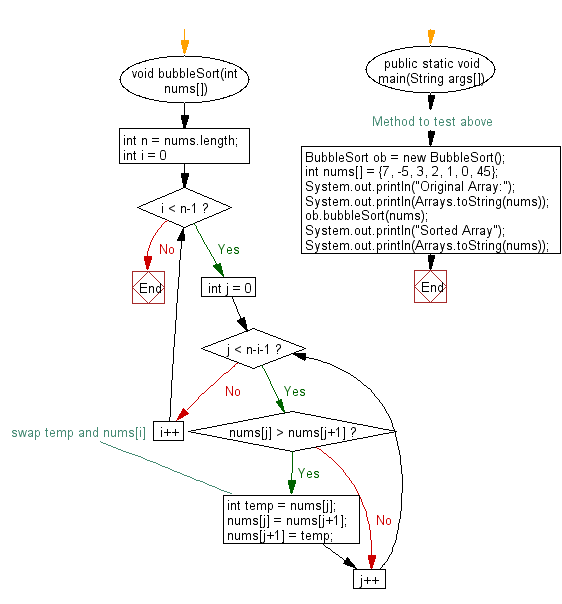
For more Practice: Solve these Related Problems:
- Write a Java program to implement bubble sort and count the number of swaps performed during sorting.
- Write a Java program to optimize bubble sort by stopping early if no swaps occur in a full pass.
- Write a Java program to modify bubble sort to sort an array of strings in lexicographical order ignoring case.
- Write a Java program to implement bubble sort in reverse order to sort an array in descending order.
Go to:
PREV : Quick Sort Implementation.
NEXT : Radix Sort Implementation.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.