Java Program: Calculate Average of Integers using Streams
Write a Java program to calculate the average of a list of integers using streams.
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List < Integer > nums = Arrays.asList(1, 3, 6, 8, 10, 18, 36);
System.out.println("List of numbers: " + nums);
// Calculate the average using streams
double average = nums.stream()
.mapToDouble(Integer::doubleValue)
.average()
.orElse(0.0);
System.out.println("Average value of the said numbers: " + average);
}
}
Sample Output:
List of numbers: [1, 3, 6, 8, 10, 18, 36] Average value of the said numbers: 11.714285714285714
Explanation:
In the above exercise, we have a list of integers (1, 3, 6, 8, 10, 18, 36). Using streams, we convert each integer to a double using the mapToDouble method and calculate the average through the average() method, and finally retrieve the average value using the orElse method in case the stream is empty. The average value is then printed to the console.
Flowchart:
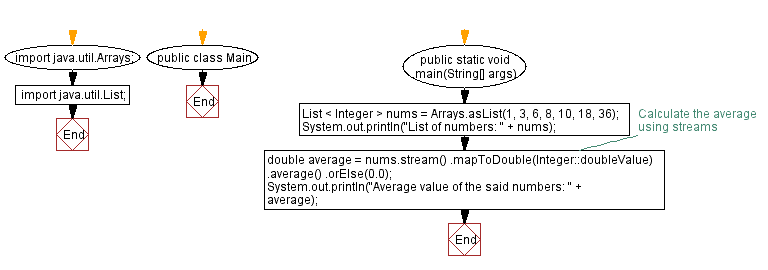
For more Practice: Solve these Related Problems:
- Write a Java program to calculate the weighted average of a list of integers using streams, where each integer is weighted by its index.
- Write a Java program to compute the average of even numbers in a list using streams and subtract it from the average of odd numbers.
- Write a Java program to calculate the average of prime numbers from a list of integers using streams.
- Write a Java program to compute the average of a list of integers using streams after filtering out numbers exceeding a given threshold.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java streams Exercises Home.
Next: Convert List of Strings to Uppercase or Lowercase using Streams.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.