Java Program: Convert List of Strings to Uppercase or Lowercase using Streams
2. Convert strings to upper/lowercase using streams
Write a Java program to convert a list of strings to uppercase or lowercase using streams.
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List < String > colors = Arrays.asList("RED", "grEEn", "white", "Orange", "pink");
System.out.println("List of strings: " + colors);
// Convert strings to uppercase using streams
List < String > uppercaseStrings = colors.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println("\nUppercase Strings: " + uppercaseStrings);
// Convert strings to lowercase using streams
List < String > lowercaseStrings = colors.stream()
.map(String::toLowerCase)
.collect(Collectors.toList());
System.out.println("Lowercase Strings: " + lowercaseStrings);
}
}
Sample Output:
List of strings: [RED, grEEn, white, Orange, pink] Uppercase Strings: [RED, GREEN, WHITE, ORANGE, PINK] Lowercase Strings: [red, green, white, orange, pink]
Explanation:
In the above exercises, we have a list of strings containing ("RED", "grEEn", "white", "Orange", "pink"). Using streams. First convert the strings to uppercase by calling the toUpperCase method on each string, and then collect the results into a new list using the collect method. Similarly, convert the strings to lowercase by calling the toLowerCase method on each string. Finally, the uppercase and lowercase lists are printed to the console.
Flowchart:
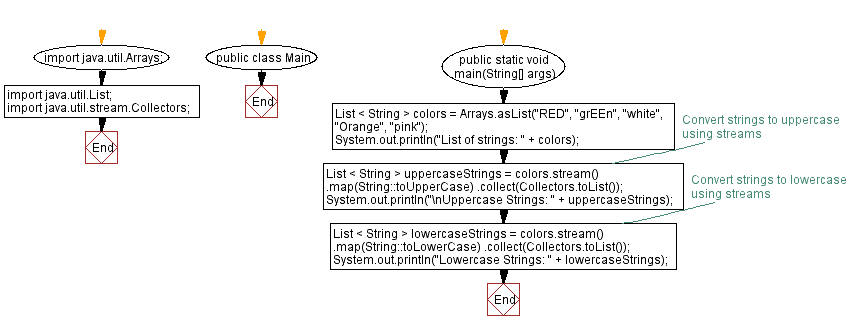
For more Practice: Solve these Related Problems:
- Write a Java program to convert a list of strings to uppercase using streams and then join them into a single comma-separated string.
- Write a Java program to convert a list of strings to lowercase using streams and sort them by their lengths.
- Write a Java program to process a list of mixed-case strings with streams to produce two lists: one entirely uppercase and one entirely lowercase.
- Write a Java program to convert each string in a list to uppercase if it starts with a vowel and to lowercase otherwise, using streams.
Go to:
PREV : Calculate average of integers using streams.
NEXT : Sum even and odd numbers in list using streams.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.