JavaScript - Check if kth bit is set or not for a number
JavaScript Bit Manipulation: Exercise-11 with Solution
Check kth Bit
Write a JavaScript program to check whether the kth bit is set or not in a given number. Return true if the kth bit is set otherwise false.
In a binary representation the presence of a non-zero value indicates that the k'th bit is set.
Test Data:
(33, 1) -> true
Explanation:
Binary format of 33 is -> 100001
K =1 -> kth bit is 1 [Non-zero value indicates that the k'th bit is set.]
(33, 2) -> false
Explanation:
Binary format of 33 is -> 100001
K =2 -> kth bit is 0 [Zero value indicates that the k'th bit is not set.]
Sample Solution:
JavaScript Code:
// Define a function to check if the kth bit of a number is set or not
const turn_On_Kth_Bit = (n, k) => {
// Check if the input is not a number
if (typeof n != "number") {
return 'It must be number!'; // Return an error message
}
// Return true if the kth bit is set, false otherwise
return (n & (1 << (k - 1))) != 0;
}
// Initialize variables n and k with values
let n = 33;
let k = 1;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
console.log("k = " + k);
// Call the turn_On_Kth_Bit function to check if the kth bit is set
let result_n = turn_On_Kth_Bit(n, k);
console.log("Check kth bit is set or not in the said number! " + result_n);
// Update variable k with a new value
k = 2;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
console.log("k = " + k);
// Call the turn_On_Kth_Bit function to check if the kth bit is set
result_n = turn_On_Kth_Bit(n, k);
console.log("Check kth bit is set or not in the said number! " + result_n);
Output:
33 in binary is 100001 k = 1 Check kth bit is set or not in the said number! true 33 in binary is 100001 k = 2 Check kth bit is set or not in the said number! false
Flowchart:
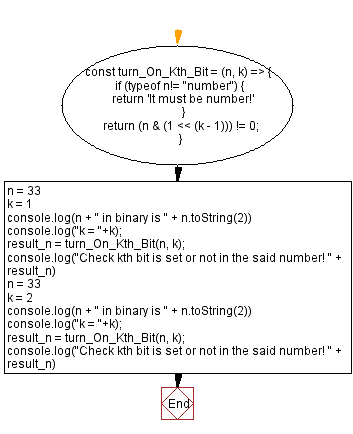
Live Demo:
See the Pen javascript-bit-manipulation-exercise-11 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if the kth bit in a given number is set by using bitwise AND with a shifted mask.
- Write a JavaScript function that returns true if the kth bit is 1, otherwise false, and validates the index.
- Write a JavaScript function that converts the number to a binary string and checks the kth character for "1".
- Write a JavaScript function that handles negative numbers by taking the absolute value before bit-checking.
Improve this sample solution and post your code through Disqus.
Previous: Turn on the kth bit of a given number.
Next: Position of the rightmost set bit of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.