JavaScript - Turn on the kth bit of a given number
JavaScript Bit Manipulation: Exercise-10 with Solution
Turn On kth Bit
Write a JavaScript program to turn on the kth bit of a given number. Return the updated number.
Test Data:
(33, 3)-> 37
Explanation:
Binary format of 33 is -> 100001
Fortmat of the said number after on the 3rd bit -> 100101
After converting 100101 to decimal : 100101 -> 37
(101, 5) -> 117
Explanation:
Binary format of 100 is -> 1100101
Fortmat of the said number after on the 5th bit -> 1110101
After converting 1110101 to decimal : 1110101 -> 117
Sample Solution:
JavaScript Code:
// Define a function to turn on the kth bit of a number
const turn_On_Kth_Bit = (n, k) => {
// Check if the input is not a number
if (typeof n != "number") {
return 'It must be number!'; // Return an error message
}
// Return the result of performing a bitwise OR operation with the kth bit
return n | (1 << (k - 1));
}
// Initialize variables n and k with values
let n = 33;
let k = 3;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
console.log("Turning k'th bit on," + " k = " + k);
// Call the turn_On_Kth_Bit function and display the result
let result_n = turn_On_Kth_Bit(n, k);
console.log(result_n + " in binary is " + result_n.toString(2));
// Update variables n and k with new values
n = 101;
k = 5;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
console.log("Turning k'th bit on," + " k = " + k);
// Call the turn_On_Kth_Bit function and display the result
result_n = turn_On_Kth_Bit(n, k);
console.log(result_n + " in binary is " + result_n.toString(2));
Output:
33 in binary is 100001 Turning k'th bit on, k = 3 37 in binary is 100101 101 in binary is 1100101 Turning k'th bit on, k = 5 117 in binary is 1110101
Flowchart:
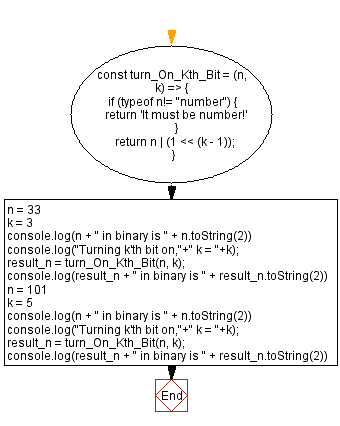
Live Demo:
See the Pen javascript-bit-manipulation-exercise-10 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that turns on (sets) the kth bit of a given number using bitwise OR with a mask.
- Write a JavaScript function that validates that the kth bit is within range before setting it.
- Write a JavaScript function that demonstrates the change by outputting the binary strings of the original and modified numbers.
- Write a JavaScript function that handles edge cases such as when the kth bit is already set.
Improve this sample solution and post your code through Disqus.
Previous: Turn off the kth bit of a given number.
Next: Check if kth bit is set or not for a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.