JavaScript - Turn off the kth bit of a given number
JavaScript Bit Manipulation: Exercise-9 with Solution
Turn Off kth Bit
Write a JavaScript program to turn off the kth bit in a given number. Return the updated number.
Test Data:
(30, 3)-> 26
Explanation:
Binary format of 30 is -> 11110
Fortmat of the said number after off the 3rd bit -> 11010
After converting 11010 to decimal : 11010 -> 26
(100, 6) -> 68
Explanation:
Binary format of 100 is -> 1100100
Fortmat of the said number after off the 6th bit -> 1000100
After converting 1000100 to decimal : 1000100 -> 68
Sample Solution:
JavaScript Code:
// Define a function to turn off the kth bit of a number
const turn_Off_Kth_Bit = (n, k) => {
// Check if the input is not a number
if (typeof n != "number") {
return 'It must be number!'; // Return an error message
}
// Return the result of performing a bitwise AND operation with the complement of the kth bit
return n & ~(1 << (k - 1));
}
// Initialize variables n and k with values
let n = 30;
let k = 3;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
console.log("Turning k'th bit off," + " k = " + k);
// Call the turn_Off_Kth_Bit function and display the result
let result_n = turn_Off_Kth_Bit(n, k);
console.log(result_n + " in binary is " + result_n.toString(2));
// Update variables n and k with new values
n = 100;
k = 6;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
console.log("Turning k'th bit off," + " k = " + k);
// Call the turn_Off_Kth_Bit function and display the result
result_n = turn_Off_Kth_Bit(n, k);
console.log(result_n + " in binary is " + result_n.toString(2));
Output:
30 in binary is 11110 Turning k'th bit off, k = 3 26 in binary is 11010 100 in binary is 1100100 Turning k'th bit off, k = 6 68 in binary is 1000100
Flowchart:
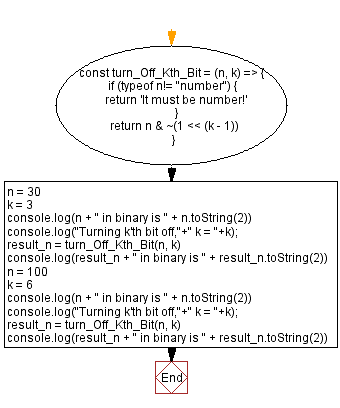
Live Demo:
See the Pen javascript-bit-manipulation-exercise-9 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that turns off (clears) the kth bit of a given integer using bitwise AND with a negated mask.
- Write a JavaScript function that validates the input and returns an error if k is not a valid bit position.
- Write a JavaScript function that displays the binary representation before and after turning off the kth bit.
- Write a JavaScript function that uses left shift to generate the mask for turning off a specified bit.
Go to:
PREV : Binary Logarithm (log2n).
NEXT : Turn On kth Bit.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.