JavaScript - Parity of a given number
JavaScript Bit Manipulation: Exercise-13 with Solution
Parity Check
Write a JavaScript program to calculate and find the parity of a given number.
In mathematics, parity is the property of an integer of whether it is even or odd. In binary numbers, parity refers to the total number of 1s. The odd parity(1) represents an odd number of 1s, whereas the even parity(0) represents an even number of 1s.
In information theory, a parity bit appended to a binary number provides the simplest form of error detecting code. If a single bit in the resulting value is changed, then it will no longer have the correct parity: changing a bit in the original number gives it a different parity than the recorded one, and changing the parity bit while not changing the number it was derived from again produces an incorrect result. In this way, all single-bit transmission errors may be reliably detected. Some more sophisticated error detecting codes are also based on the use of multiple parity bits for subsets of the bits of the original encoded value.
Test Data:
(34) -> "Parity of 34 is even."
"34 in binary is 100010" // Even number of 1s
(104) -> "Parity of 104 is odd."
"104 in binary is 1101000" // Odd number of 1s
Sample Solution:
JavaScript Code:
// Define a function to determine the parity (even or odd) of a number
const parity_even_odd = (n) => {
let parity = false; // Initialize parity as false (even)
let temp = n; // Store the original value of n in a temporary variable
while (n != 0) {
// Check if the least significant bit (LSB) of n is 1
if ((n & 1) !== 0) {
parity = !parity; // Flip the parity if LSB is 1
}
n = n >> 1; // Right shift n by 1 bit
}
// Return a message indicating whether the parity is even or odd
if (parity) {
return "Parity of " + temp + " is odd.";
} else {
return "Parity of " + temp + " is even.";
}
}
// Initialize variable n with a value
let n = 34;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
// Call the parity_even_odd function to determine the parity of n
console.log(parity_even_odd(n));
// Update variable n with a new value
n = 104;
// Display the binary representation of n
console.log(n + " in binary is " + n.toString(2));
// Call the parity_even_odd function to determine the parity of n
console.log(parity_even_odd(n));
Output:
34 in binary is 100010 Parity of 34 is even. 104 in binary is 1101000 Parity of 104 is odd.
Flowchart:
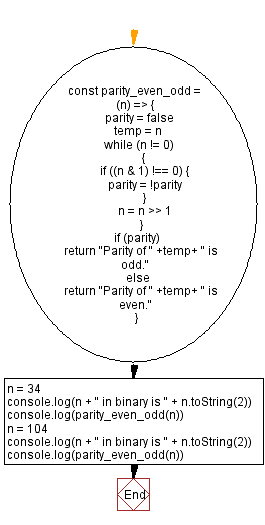
Live Demo:
See the Pen javascript-bit-manipulation-exercise-12 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the parity (even or odd count of 1s) of a number's binary representation using bitwise operators.
- Write a JavaScript function that iterates through the bits of a number and counts the 1s to determine if the count is even or odd.
- Write a JavaScript function that uses recursion or a loop to compute the parity of a given integer.
- Write a JavaScript function that returns a string stating "Parity of X is even/odd" based on the bit count.
Improve this sample solution and post your code through Disqus.
Previous: Position of the rightmost set bit of a number.
Next: Find the non-repeated element from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.