JavaScript - Swap two variables using bit manipulation
JavaScript Bit Manipulation: Exercise-2 with Solution
Write a JavaScript program to swap two variables using bit manipulation.
Test Data:
(12, 15) -> (15,12)
Sample Solution:
JavaScript Code:
// Define a function to swap the values of two variables using bitwise XOR operations
const swap = (x, y) => {
x = x ^ y // Perform bitwise XOR to swap values
y = x ^ y // Perform bitwise XOR to swap values
x = x ^ y // Perform bitwise XOR to swap values
return {a:x, b:y} // Return an object with the swapped values
}
x = 12 // Assign a value to variable x
y = 15 // Assign a value to variable y
console.log("Before swap: x = " + x + " and y = " + y) // Print the values of x and y before swapping
const {a,b} = swap(x, y) // Call the swap function and destructure the returned object
console.log("After swap: x = " + a + " and y = " + b) // Print the values of x and y after swapping
Output:
Before swap: x = 12 and y = 15 After swap: x = 15 and y = 12
Flowchart:
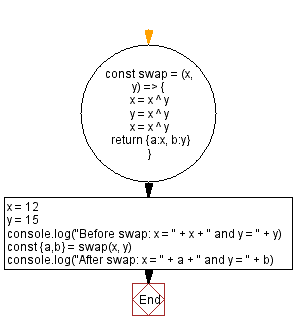
Live Demo:
See the Pen javascript-bit-manipulation-exercise-1 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
Improve this sample solution and post your code through Disqus.
Previous: Check two integers have opposite signs or not.
Next: Number of 0 bits in a binary representation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics