JavaScript - Odd or even number using bit manipulation
JavaScript Bit Manipulation: Exercise-5 with Solution
Odd or Even (Bits)
Write a JavaScript program to check if a given number is odd or even using bit manipulation.
A number (i.e., integer) expressed in the decimal numeral system is even or odd according to whether its last digit is even or odd. That is, if the last digit is 1, 3, 5, 7, or 9, then it is odd; otherwise it is even-as the last digit of any even number is 0, 2, 4, 6, or 8.
Test Data:
(1) -> 1 is an odd number.
(4) -> 4 is an even number.
(9) -> 9 is an odd number.
("15") -> Parameter value must be number!
Sample Solution:
JavaScript Code:
// Define a function to check whether a number is even or odd
const check_even_odd = (n) => {
// Check if the input is not a number
if (typeof n != "number")
{
return 'Parameter value must be number!' // Return an error message
}
// Check if the number is even
if ((n ^ 1) == (n + 1))//even
return n +' is an even number.' // Return a message indicating that the number is even
else //odd
return n +' is an odd number.' // Return a message indicating that the number is odd
}
console.log(check_even_odd(1)) // Test the function with the number 1
console.log(check_even_odd(4)) // Test the function with the number 4
console.log(check_even_odd(9)) // Test the function with the number 9
console.log(check_even_odd("15")) // Test the function with the string "15"
Output:
1 is an odd number. 4 is an even number. 9 is an odd number. Parameter value must be number!
Flowchart:
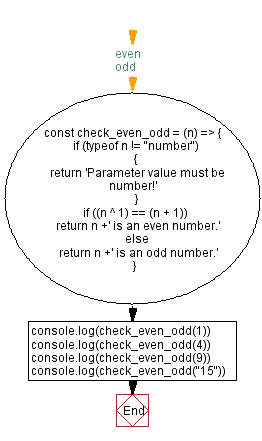
Live Demo:
See the Pen javascript-bit-manipulation-exercise-5 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
For more Practice: Solve these Related Problems:
- Write a JavaScript function that determines if a number is odd or even using the bitwise AND operator.
- Write a JavaScript function that checks the least significant bit of a number to return "odd" or "even".
- Write a JavaScript function that validates input type and returns an error for non-numeric values.
- Write a JavaScript function that uses bit shifting to isolate the last bit and decide the number's parity.
Improve this sample solution and post your code through Disqus.
Previous: Next power of two of a given number.
Next: Check a number is a power of 4 or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.