JavaScript - Check a number is a power of 4 or not
JavaScript Bit Manipulation: Exercise-6 with Solution
Write a JavaScript program to check if a given positive number is a power of four or not using bit manipulation.
The expression n & (n-1) will unset the rightmost set bit of a number. If the number is a power of 2, it has only a 1-bit set, and n & (n-1) will unset the only set bit. So, we can say that n & (n-1) returns 0 if n is a power of 2; otherwise, it's not a power of 2.
The mask 0xAAAAAAAA will allow us to determine where the set bit is located. In all of its odd positions, the mask 0xAAAAAAAA has a value of 1. The set bit in n will be even if the expression (n & 0xAAAAAAAA) is true.
(0xAAAAAAAA)16 = (10101010101010101010101010101010)2.
Test Data:
(256) -> true
(4) -> true
(2) -> false
("16") -> "It must be number!"
Sample Solution:
JavaScript Code:
// Define a function to check if a number is a power of four
const Power_of_four = (n) => {
// Check if the input is not a number
if (typeof n!= "number") {
return 'It must be number!' // Return an error message
}
// Check if the number is not zero, is a power of two, and has only one bit set in even position
return n != 0 && (n & (n - 1)) == 0 && (n & 0xAAAAAAAA) == 0;
}
console.log(Power_of_four(256)) // Test the function with the number 256
console.log(Power_of_four(4)) // Test the function with the number 4
console.log(Power_of_four(2)) // Test the function with the number 2
console.log(Power_of_four("16")) // Test the function with the string "16"
Output:
true true false It must be number!
Flowchart:
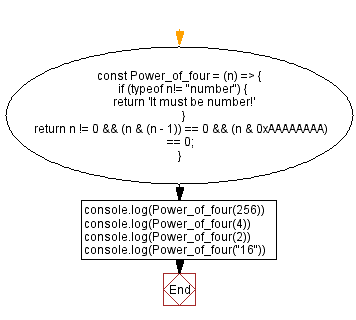
Live Demo:
See the Pen javascript-bit-manipulation-exercise-6 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
Improve this sample solution and post your code through Disqus.
Previous: Odd or even number using bit manipulation.
Next: Swap two bits at given position in an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics