JavaScript Function: Validate integer parameters with custom Error
JavaScript Error Handling: Exercise-1 with Solution
Throw Error for Non-Integer
Write a JavaScript function that takes a number as a parameter and throws a custom 'Error' if the number is not an integer.
Sample Solution:
JavaScript Code:
// Define a function named validateInteger which takes a parameter 'number'
function validateInteger(number)
{
// Check if the given number is not an integer using Number.isInteger() method
if (!Number.isInteger(number))
{
// If the number is not an integer, throw an error with a specific message
throw new Error('Invalid number. Please input an integer.');
}
// Log a message indicating that the number is valid
console.log('Number is valid:', number);
}
// Example usage:
try {
// Call validateInteger function with an integer argument (12)
validateInteger(12); // Valid integer
// Call validateInteger function with a non-integer argument (2.12)
validateInteger(2.12); // Throws an error
} catch (error) {
// Catch any errors thrown by the validateInteger function and log the error message
console.log('Error:', error.message);
}
Output:
"Number is valid:" 12 "Error:" "Invalid number. Please input an integer."
Note: Executed on JS Bin
Explanation:
In the above function, the "Number.isInteger()" method is used to check if the given number is an integer. If it's not an integer, the program throws a custom "Error" with the message "Invalid number. Please input an integer." If the number is valid, the program logs a success message.
In the example usage, we demonstrate how the function works by calling it with both a valid integer (42) and an invalid number (3.14). The try-catch block catches and handles any thrown error, and the error message is logged to the console.
Flowchart:
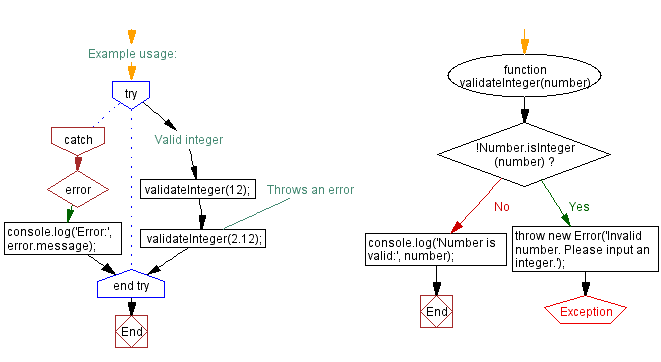
Live Demo:
See the Pen javascript-error-handling-exercise-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: JavaScript Error Handling Exercises Home.
Error Handling Exercises Next: Handling TypeError with Try-Catch block.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/error-handling/javascript-error-handling-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics