JavaScript Program: Handling TypeError with Try-Catch block
JavaScript Error Handling: Exercise-2 with Solution
Write a JavaScript program that uses a try-catch block to catch and handle a 'TypeError' when accessing a property of an undefined object.
Sample Solution:
JavaScript Code:
// Try block to handle potential errors
try {
// Declare a constant variable undefinedObject and assign it the value undefined
const undefinedObject = undefined;
// Access a property of the undefined object, which will result in an error
console.log(undefinedObject.property); // Accessing property of undefined object
}
// Catch block to handle errors thrown in the try block
catch (error) {
// Check if the error is an instance of TypeError
if (error instanceof TypeError)
{
// Log an error message indicating property access to an undefined object
console.log('Error: Property access to undefined object');
}
// If the error is not a TypeError, rethrow the error
else
{
throw error; // Rethrow the error if it's not a TypeError
}
}
Output:
"Error: Property access to undefined object"
Note: Executed on JS Bin
Explanation:
In the above exercise, we create a variable undefinedObject and assign it the value of undefined. Then, we try to access undefinedObject's property. Since undefined has no properties, this operation will result in a TypeError.
The code is wrapped in a try-catch block. Inside the catch block, we check if the caught error is an instance of TypeError using the instanceof operator. If it is, we log the custom error message 'Error: Property access to undefined object'. Otherwise, if the error is not a TypeError, it might be a different type of error, so we rethrow the error using throw error.
Flowchart:
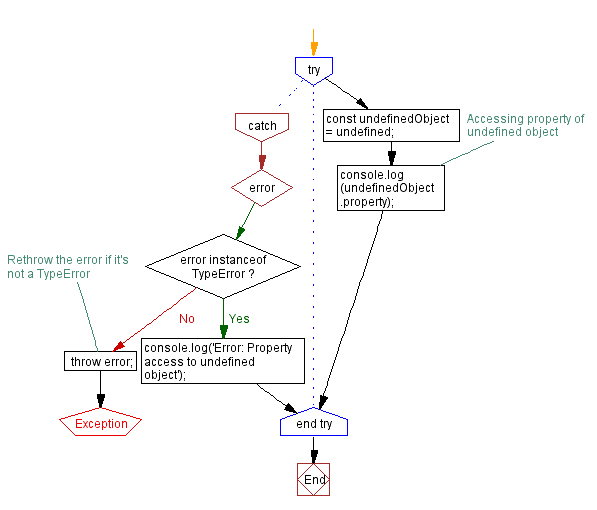
Live Demo:
See the Pen javascript-error-handling-exercise-2 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Validate integer parameters with custom Error.
Error Handling Exercises Next: Custom Error on the second number zero.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics