JavaScript Program: Catching and handling ReferenceError with the try-catch Block
JavaScript Error Handling: Exercise-12 with Solution
Handle ReferenceError
Write a JavaScript program that uses a try-catch block to catch and handle a 'ReferenceError' when accessing an undefined variable.
Sample Solution:
JavaScript Code:
// Define a function named access_Variable that attempts to access an undefined variable
function access_Variable() {
// Try block to attempt accessing the undefined variable and handle potential errors
try {
// Attempt to log the value of an undefined variable to the console
console.log(undefinedVariable);
} catch (error) {
// Catch block to handle errors
// Check if the error is an instance of ReferenceError
if (error instanceof ReferenceError) {
// If the error is a ReferenceError, log the error message to the console
console.log('ReferenceError:', error.message);
} else {
// If the error is not a ReferenceError, log the error message to the console
console.log('Error:', error.message);
}
}
}
// Example:
// Call the access_Variable function to demonstrate accessing an undefined variable
access_Variable();
Output:
"ReferenceError:" "undefinedVariable is not defined"
Note: Executed on JS Bin
Explanation:
In the above exercise,
- Inside the try block, the console.log(undefinedVariable) statement throws a ReferenceError because the variable does not exist. The code then jumps to the catch block.
- In the catch block, the error object is caught in the error parameter. The code checks if the error is an instance of ReferenceError using the instanceof operator. If it is, the error message is logged to the console as 'ReferenceError: <error message>'. If it's a different type of error, the error message is logged as 'Error: <error message>'.
- In the example calling access_Variable() triggers a ReferenceError because undefinedVariable is not defined. The error message, 'ReferenceError: undefinedVariable is not defined', is logged to the console.
Flowchart:
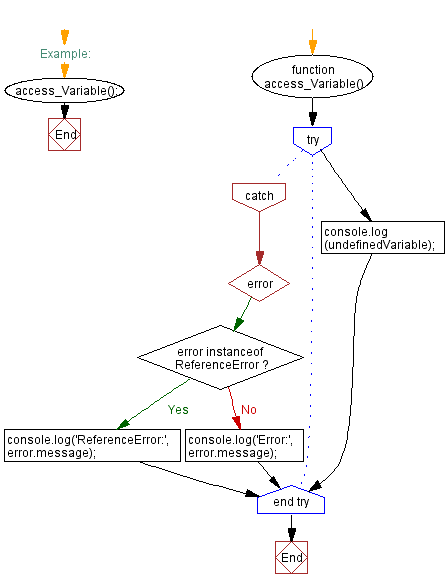
Live Demo:
See the Pen javascript-error-handling-exercise-12 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that attempts to access an undeclared variable and catches the resulting ReferenceError.
- Write a JavaScript function that dynamically evaluates code with a missing variable reference and handles the ReferenceError accordingly.
- Write a JavaScript function that simulates the use of an uninitialized variable and demonstrates ReferenceError handling with try-catch.
- Write a JavaScript function that checks for the existence of a variable and deliberately triggers a ReferenceError to test error recovery.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Catching and handling EvalError with the try-catch Block.
Error Handling Exercises Next: Catching and handling JSON string SyntaxError.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.