JavaScript Program: Catching and handling SyntaxError with the try-catch block
JavaScript Error Handling: Exercise-13 with Solution
Write a JavaScript program that uses a try-catch block to catch and handle a 'SyntaxError' when parsing an invalid JSON string.
Sample Solution:
JavaScript Code:
// Define a function named parse_JSON that attempts to parse a JSON string
function parse_JSON(jsonString) {
// Try block to attempt parsing the JSON string and handle potential errors
try {
// Attempt to parse the JSON string and store the parsed data
const parsedData = JSON.parse(jsonString);
// Log the parsed data to the console
console.log('Parsed data:', parsedData);
} catch (error) {
// Catch block to handle errors
// Check if the error is an instance of SyntaxError
if (error instanceof SyntaxError) {
// If the error is a SyntaxError, log the error message to the console
console.log('SyntaxError:', error.message);
} else {
// If the error is not a SyntaxError, log the error message to the console
console.log('Error:', error.message);
}
}
}
// Example:
// Call the parse_JSON function to demonstrate parsing JSON strings
parse_JSON('{"name": "Rowan Octave", "age": 30}'); // Valid JSON
parse_JSON('{"name": "Rowan Octave", "age": 30,}'); // Invalid JSON
Output:
"Parsed data:" [object Object] { age: 30, name: "Rowan Octave" } "SyntaxError:" "Expected double-quoted property name in JSON at position 35"
Note: Executed on JS Bin
Explanation:
In the above exercise -
The "parse_JSON()" function parses a JSON string using JSON.parse(jsonString). If the JSON string is valid, the parsed data is logged to the console.
Inside the try block, the JSON.parse function is called with the 'jsonString' parameter. If the JSON string is well-formed, parsing is successful, and the parsed data is stored in the 'parsedData' variable. The code then logs 'Parsed data: <parsed data>' to the console.
If the JSON string is not valid and results in a syntax error, the code jumps to the catch block.
In the catch block, the error object is caught in the error parameter. The code checks if the error is an instance of 'SyntaxError' using the 'instanceof' operator. If it is, the error message is logged to the console as 'SyntaxError: <error message>'. If it's a different type of error, the error message is logged as 'Error: <error message>'.
Flowchart:
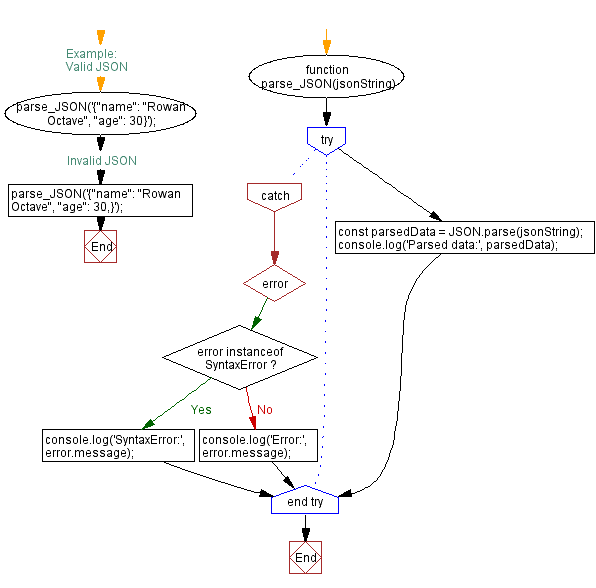
Live Demo:
See the Pen javascript-error-handling-exercise-13 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Error Handling Exercises Previous: Catching and handling ReferenceError with the try-catch Block.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics