JavaScript button click event listener - Interactive program
JavaScript Event Handling: Exercise-1 with Solution
Button Click Event
Write a JavaScript program that creates a button and add a click event listener to log a message when it's clicked.
Sample Solution:
JavaScript Code:
// Create a button element
const button = document.createElement('button');
button.textContent = 'Click me';
// Add click event listener to the button
button.addEventListener('click', () => {
console.log('Button clicked!');
});
// Append the button to the document body
document.body.appendChild(button);
Output:
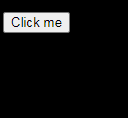
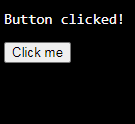
Note: Executed on JS Bin
Explanation:
First, we create a new button element using the "createElement()" method and set its text content to "Click me". We then add a click event listener to the button using the "addEventListener()" method. When the button is clicked, the callback function inside the event listener is executed, which logs the message "Button clicked!" to the console.
Finally, we append the button to the document body using the "appendChild()" method so that it becomes visible on the page.
Flowchart:
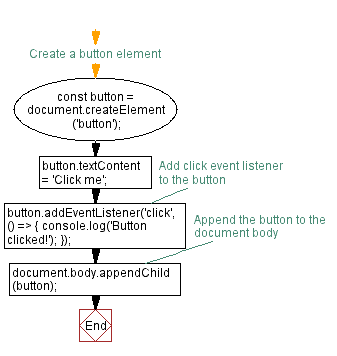
Live Demo:
See the Pen javascript-event-handling-exercise-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that dynamically creates multiple buttons and attaches a click event to each, logging its index when clicked.
- Write a JavaScript function that toggles a CSS class on a button upon each click and logs the updated class name.
- Write a JavaScript program that changes a button’s text each time it is clicked and records the number of clicks.
- Write a JavaScript function that attaches a one-time click event to a button, ensuring the event listener is removed after the first click.
Go to:
PREV : JavaScript Event Handling Exercises Home.
NEXT : Dropdown Menu Toggle.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.