JavaScript Dropdown menu - Interactive program
JavaScript Event Handling: Exercise-2 with Solution
Write a JavaScript program to create a dropdown menu that shows and hides its options when clicked.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<style>
.dropdown {
position: relative;
display: inline-block;
}
.dropdown-button {
background-color:#7FFFD4;
color: #333;
padding: 10px;
border: none;
cursor: pointer;
}
.dropdown-options {
display: none;
position: absolute;
background-color: #CC56FF;
min-width: 120px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
padding: 0;
margin: 0;
list-style: none;
z-index: 1;
}
.dropdown-option {
padding: 10px;
cursor: pointer;
border-bottom: 1px solid #ccc;
}
.dropdown-option:last-child {
border-bottom: none;
}
</style>
</head>
<body>
<div class="dropdown">
<button class="dropdown-button">Select a Subject</button>
<ul id="dropdown-options" class="dropdown-options">
<li class="dropdown-option">Mathematics</li>
<li class="dropdown-option">English</li>
<li class="dropdown-option">Physics</li>
</ul>
</div>
<script>
const dropdownButton = document.querySelector('.dropdown-button');
const dropdownOptions = document.querySelector('.dropdown-options');
dropdownButton.addEventListener('click', () => {
dropdownOptions.style.display = dropdownOptions.style.display === 'none' ? 'block' : 'none';
});
</script>
</body>
</html>
Output:
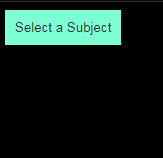
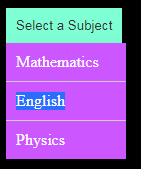
Note: Executed on JS Bin
Explanation:
In the above exercise -
- First the HTML document creates a dropdown menu with three options: Mathematics, English, and Physics. Here's an explanation of the code:
- The <style> section contains CSS styles for the dropdown and its options.
- Inside the <body> section, there is a <div> element with the class "dropdown" that acts as a container for the dropdown menu.
- Inside the <div>, there is a <button> element with the class "dropdown-button". This button is the visible part of the dropdown menu that users can click to show or hide the options.
- Below the button, there is a <ul> element with the id "dropdown-options" and the class "dropdown-options". This is an unordered list of dropdown options.
- Inside the <ul>, there are three <li> elements with the class "dropdown-option". These list items represent the available options in the dropdown menu.
- In the <script> section, JavaScript code is used to add functionality to the dropdown menu. It selects the dropdown button and dropdown options using document.querySelector() and assigns them to the dropdownButton and dropdownOptions variables.
- An event listener is added to the dropdown button using addEventListener(). When the button is clicked, the event listener function is executed.
Flowchart:
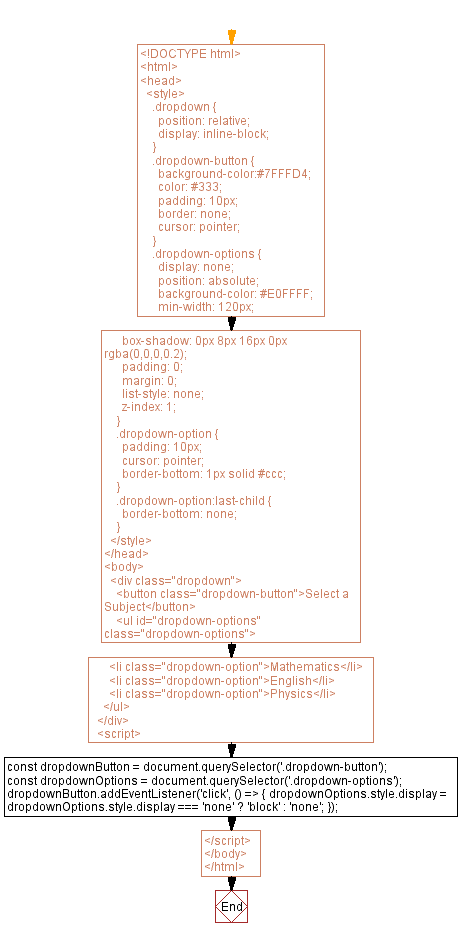
Live Demo:
See the Pen javascript-event-handling-exercise-2 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript button click event listener - Interactive program.
Event Handling Exercises Next: JavaScript mouse enter event - Change background color.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics