JavaScript mouse enter event - Change background color
JavaScript Event Handling: Exercise-3 with Solution
Write a JavaScript function that changes the background color of an element when a mouse enters it.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<style>
.my-element {
width: 200px;
height: 200px;
background-color: lightgray;
}
</style>
</head>
<body>
<div id="myDiv" class="my-element"></div>
<script>
function changeBackgroundColor(elementId, color) {
const element = document.getElementById(elementId);
if (element) {
element.addEventListener('mouseenter', () => {
element.style.backgroundColor = color;
});
}
}
// Call the function with element ID and desired color
changeBackgroundColor('myDiv', 'green');
</script>
</body>
</html>
Output:
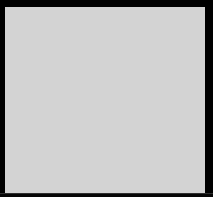
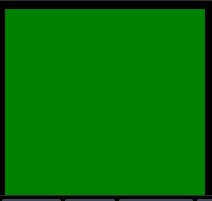
Note: Executed on JS Bin
Explanation:
In the above exercise -
- The "changeBackgroundColor()" function takes two parameters: elementId and color. The elementId is the ID of the element you want to target, and color is the desired background color.
- Inside the function, it first retrieves the element using document.getElementById() and assigns it to the element variable.
- Event listeners are added to the element using addEventListener(). The event being listened to is 'mouseenter', which triggers when the mouse enters the element.
- When the 'mouseenter' event is triggered, the callback function sets the backgroundColor CSS property of the element to the specified color value.
Flowchart:
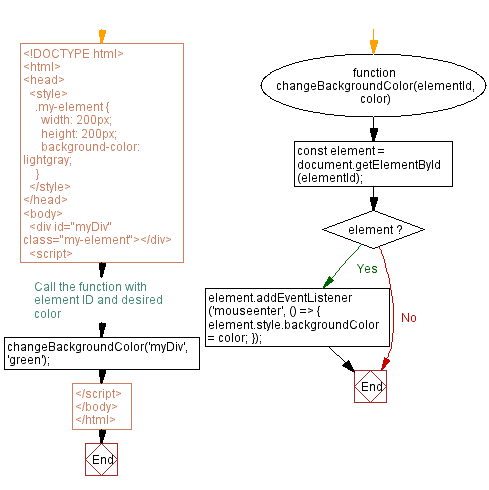
Live Demo:
See the Pen javascript-event-handling-exercise-3 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript Dropdown menu - Interactive program.
Event Handling Exercises Next: JavaScript form validation - Display error for empty fields.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics