JavaScript form validation - Display error for empty fields
JavaScript Event Handling: Exercise-4 with Solution
Write a JavaScript program that implements a "form" validation that displays an error message if a required field is left empty when submitting the form.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<style>
.error-message {
color: red;
margin-top: 5px;
}
</style>
</head>
<body>
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" required>
<br>
<label for="email">Email:</label>
<input type="email" id="email" required>
<br>
<label for="message">Message:</label>
<textarea id="message" required></textarea>
<br>
<button type="submit">Submit</button>
</form>
<div id="errorMessages"></div>
<script>
const form = document.getElementById('myForm');
const errorMessagesDiv = document.getElementById('errorMessages');
form.addEventListener('submit', (event) => {
event.preventDefault();
errorMessagesDiv.innerHTML = '';
const requiredFields = form.querySelectorAll('[required]');
requiredFields.forEach((field) => {
if (field.value.trim() === '') {
const fieldName = field.getAttribute('name');
const errorMessage = document.createElement('p');
errorMessage.textContent = `${fieldName} is required.`;
errorMessagesDiv.appendChild(errorMessage);
}
});
});
</script>
</body>
</html>
Output:
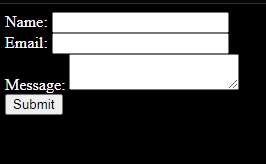
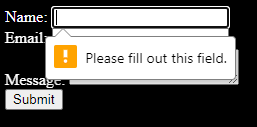
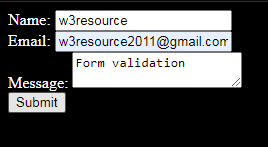
Note: Executed on JS Bin
Explanation:
In the above exercise,
- The HTML structure consists of a <form> element with an id of "myForm" that contains several input fields and a submit button. Below the form, there is a <div> element with an id of "errorMessages" which serves as a container for displaying error messages.
- The JavaScript code attaches an event listener to the form's "submit" event using addEventListener. When the form is submitted, the event listener function is triggered.
- Inside the event listener function, the default form submission behavior is prevented using event.preventDefault() to stop the form from being submitted and the page from being refreshed.
- The code then retrieves all the input fields with the required attribute using form.querySelectorAll('[required]'). It loops through each of these required fields using forEach and checks if the field's value, after trimming any leading or trailing spaces, is empty. If a required field is empty, an error message is created as a <p> element. Its content is set to indicate the field name followed by the text "is required."
- The error message element is then appended to the "errorMessagesDiv" container using appendChild.
Flowchart:
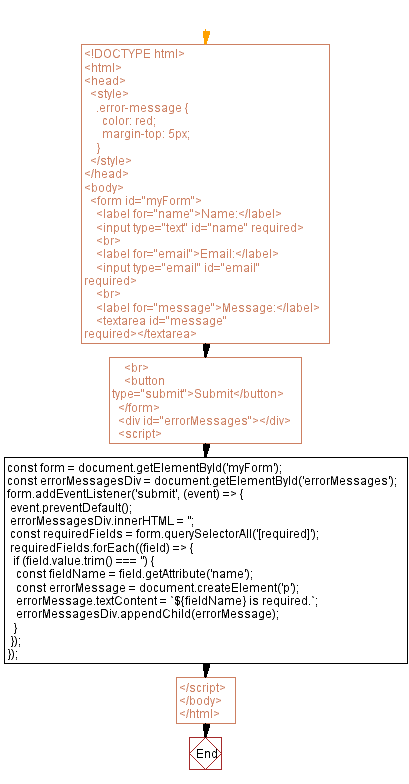
Live Demo:
See the Pen javascript-event-handling-exercise-4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript mouse enter event - Change background color.
Event Handling Exercises Next: JavaScript Slideshow - Next & previous button image change.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics