JavaScript Slideshow - Next & previous button image change
JavaScript Event Handling: Exercise-5 with Solution
Write a JavaScript program to create a slideshow that changes the displayed image when a next or previous button is clicked.
Sample Solution:
HTML and JavaScript Code:
<!DOCTYPE html>
<html>
<head>
<style>
.slideshow {
display: flex;
justify-content: center;
align-items: center;
height: 300px;
}
.slideshow img {
width: 300px;
height: 300px;
}
</style>
</head>
<body>
<div class="slideshow">
<button id="previousBtn">Previous</button>
<img id="image" src="image1.jpg">
<button id="nextBtn">Next</button>
</div>
<script>
const images = ['image1.jpg', 'image2.jpg', 'image3.jpg'];
let currentIndex = 0;
const previousBtn = document.getElementById('previousBtn');
const nextBtn = document.getElementById('nextBtn');
const image = document.getElementById('image');
previousBtn.addEventListener('click', () => {
currentIndex = (currentIndex - 1 + images.length) % images.length;
image.src = images[currentIndex];
});
nextBtn.addEventListener('click', () => {
currentIndex = (currentIndex + 1) % images.length;
image.src = images[currentIndex];
});
</script>
</body>
</html>
Output:
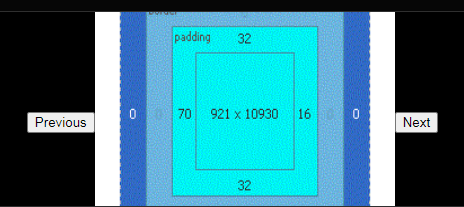
Note: Executed on JS Bin
Flowchart:
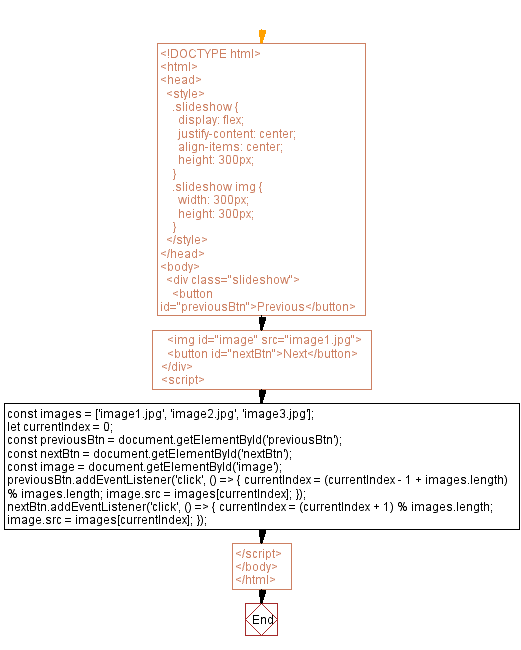
Live Demo:
See the Pen javascript-event-handling-exercise-5 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Event Handling Exercises Previous: JavaScript form validation - Display error for empty fields.
Event Handling Exercises Next: JavaScript Drag-and-Drop: Reorder items in a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics