JavaScript: Return the object associating the properties to the values of a given array of valid property identifiers and an array of values
JavaScript fundamental (ES6 Syntax): Exercise-101 with Solution
Object with Array Properties and Values
Write a JavaScript program to return the object associating the properties to the values of a given array of valid property identifiers and an array of values.
Note: Since an object can have undefined values but not undefined property pointers, the array of properties is used to decide the structure of the resulting object using Array.reduce().
Associates properties to values, given array of valid property identifiers and an array of values.
- Use Array.prototype.reduce() to build an object from the two arrays.
- If the length of props is longer than values, remaining keys will be undefined.
- If the length of values is longer than props, remaining values will be ignored.
Sample Solution:
JavaScript Code:
function zipObject(keys, values) {
return keys.reduce((acc, curr, indx) => {
if (values[indx] !== undefined) { // Ensure the value exists
const key = curr;
const val = values[indx];
acc[key] = val;
}
return acc; // Return the accumulator
}, {});
}
// Test the 'zipObject' function
console.log(zipObject(['a', 'b', 'c'], [1, 2])); // {"a":1,"b":2}
console.log(zipObject(['a', 'b'], [1, 2, 3])); // {"a":1,"b":2}
Explanation:
Function Definition:
- The function zipObject(keys, values) takes two arrays as arguments:
- keys: An array of property names.
- values: An array of corresponding values.
Reduce Method:
- keys.reduce() is used to iterate over the keys array and build the result object (acc).
- The acc parameter starts as an empty object ({}).
Check for Undefined Values:
- During each iteration, the function checks if values[indx] (the value corresponding to the current key) is not undefined.
- If the value is defined, it assigns the value to the corresponding key in the accumulator object (acc).
Assigning Key-Value Pairs:
- Inside the if block:
- key is set to the current key (curr).
- val is set to the value at the same index in the values array.
- These are then added to the acc object as a key-value pair.
Return Accumulator:
- After processing all keys, the function returns the completed accumulator object.
Test Cases:
- First Test Case:
- Input: keys = ['a', 'b', 'c'], values = [1, 2].
- The function maps:
- 'a' -> 1
- 'b' -> 2
- 'c' is skipped because values[2] is undefined.
- Output: {"a": 1, "b": 2}.
- Second Test Case:
- Input: keys = ['a', 'b'], values = [1, 2, 3].
- The function maps:
- 'a' -> 1
- 'b' -> 2.
- Any extra values in values (3 in this case) are ignored because there is no corresponding key.
- Output: {"a": 1, "b": 2}.
Output:
[object Object] { a: 1, b: 2 } [object Object] { a: 1, b: 2 }
Visual Presentation:
Flowchart:
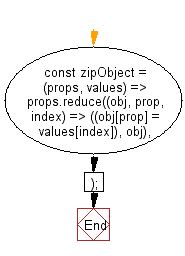
Live Demo:
See the Pen javascript-basic-exercise-101-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that takes an array of property names and an array of values to create an object mapping each name to its value.
- Write a JavaScript function that validates two arrays (keys and values) and returns an object pairing each key with the corresponding value.
- Write a JavaScript program that constructs an object from two arrays, handling cases where the arrays differ in length.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an array of elements, grouped based on the position in the original arrays and using function as the last value to specify how grouped values should be combined.
Next: Write a JavaScript program to create an array of elements, grouped based on the position in the original arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.