JavaScript : Create an array of elements, grouped based on the position in the original arrays and using function as the last value to specify how grouped values should be combined
JavaScript fundamental (ES6 Syntax): Exercise-100 with Solution
Group Array by Index and Combine with Function
Write a JavaScript program to create an array of elements, grouped based on the position in the original arrays. Use function as the last value to specify how grouped values should be combined.
Note: Check if the last argument provided is a function.
- Check if the last argument provided is a function.
- Use Math.max() to get the longest array in the arguments.
- Use Array.from() to create an array with appropriate length and a mapping function to create array of grouped elements.
- If lengths of the argument arrays vary, undefined is used where no value could be found.
- The function is invoked with the elements of each group.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'zipWith' function to zip arrays together and apply a custom function to their elements.
const zipWith = (...arrays) => {
// Extract the custom function if provided.
const fn = typeof arrays[arrays.length - 1] === 'function' ? arrays.pop() : undefined;
// Create a new array by iterating over the length of the longest array.
return Array.from(
{ length: Math.max(...arrays.map(a => a.length)) },
(_, i) => {
// Apply the custom function to the corresponding elements of the arrays, if provided.
if (fn) return fn(...arrays.map(a => a[i]));
// Otherwise, return an array containing the elements at index 'i' from each array.
return arrays.map(a => a[i]);
}
);
};
// Test the 'zipWith' function with arrays and a custom function.
console.log(zipWith(
[1, 2, 3],
[10, 20],
[100, 200],
(a, b, c) => (a != null ? a : 'a') + (b != null ? b : 'b') + (c != null ? c : 'c')
));
Output:
[111,222,"3bc"]
Flowchart:
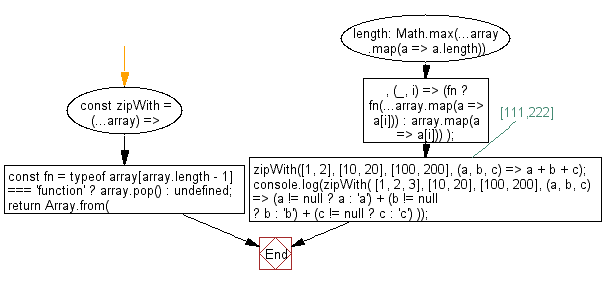
Live Demo:
See the Pen javascript-basic-exercise-100-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to hash an given input string into a whole number.
Next: Write a JavaScript program to return the object associating the properties to the values of an given array of valid property identifiers and an array of values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-100.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics