JavaScript : Hash an given input string into a whole number
JavaScript fundamental (ES6 Syntax): Exercise-99 with Solution
Hash String into Number
Write a JavaScript program to hash an given input string into a whole number.
Hashes the input string into a whole number.
- Use String.prototype.split('') and Array.prototype.reduce() to create a hash of the input string, utilizing bit shifting.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the 'sdbm' function to generate a hash code using the SDBM algorithm for a given string.
const sdbm = str => {
// Convert the string into an array of characters.
let arr = str.split('');
// Reduce the array to calculate the hash code using the SDBM algorithm.
return arr.reduce(
(hashCode, currentVal) =>
// Calculate the hash code using the SDBM algorithm.
(hashCode = currentVal.charCodeAt(0) + (hashCode << 6) + (hashCode << 16) - hashCode),
0
);
};
// Test the 'sdbm' function with sample strings.
console.log(sdbm('w3r'));
console.log(sdbm('name'));
Output:
986464758 -3521204949
Flowchart:
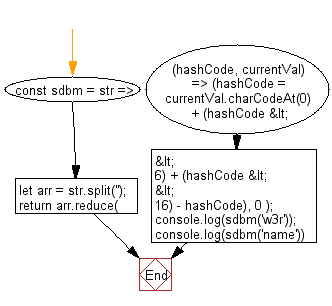
Live Demo:
See the Pen javascript-basic-exercise-99-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a string into a numeric hash using a simple hash algorithm.
- Write a JavaScript function that iterates over a string’s characters and computes a hash value based on their char codes.
- Write a JavaScript program that generates a whole number hash from an input string while handling collisions minimally.
Go to:
PREV : Filter Array of Objects by Condition.
NEXT : Group Array by Index and Combine with Function.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.