JavaScript: Get all unique values (form the right side of the array) of an array, based on a provided comparator function
JavaScript fundamental (ES6 Syntax): Exercise-107 with Solution
Distinct Values (Right-Side) by Comparator
Write a JavaScript program to get all distinct values (from the right side of the array) of an array, based on a provided comparator function.
- Use Array.prototype.reduceRight() and Array.prototype.some() for an array containing only the last unique occurrence of each value, based on the comparator function, fn.
- The comparator function takes two arguments: the values of the two elements being compared.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'uniqueElementsByRight' to return unique elements from an array based on a comparison function, iterating from right to left.
const uniqueElementsByRight = (arr, fn) =>
// Use reduceRight to iterate over the array 'arr' from right to left.
arr.reduceRight((acc, v) => {
// If the accumulator 'acc' does not contain any element that satisfies the comparison function 'fn' with the current element 'v', add 'v' to the accumulator.
if (!acc.some(x => fn(v, x))) acc.push(v);
// Return the accumulator.
return acc;
}, []);
// Test the 'uniqueElementsByRight' function with an example.
console.log(uniqueElementsByRight(
[
{ id: 0, value: 'a' },
{ id: 1, value: 'b' },
{ id: 2, value: 'c' },
{ id: 1, value: 'd' },
{ id: 0, value: 'e' }
],
(a, b) => a.id == b.id
));
Output:
[{"id":0,"value":"e"},{"id":1,"value":"d"},{"id":2,"value":"c"}]
Flowchart:
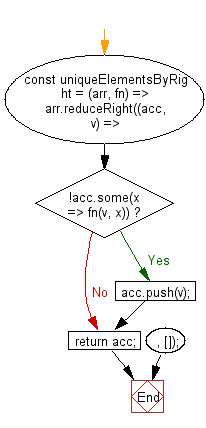
Live Demo:
See the Pen javascript-basic-exercise-107-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an array of elements, ungrouping the elements in an array produced by zip and applying the provided function.
Next: Write a JavaScript program to get all unique values of an array, based on a provided comparator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-107.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics