JavaScript: Get a random element from an array
JavaScript fundamental (ES6 Syntax): Exercise-149 with Solution
Random Element from Array
Write a JavaScript program to get a random element from an array.
- Use Math.random() to generate a random number.
- Multiply it by Array.prototype.length and round it off to the nearest whole number using Math.floor().
- This method also works with strings.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define the sample function
const sample = arr => arr[Math.floor(Math.random() * arr.length)];
// Select a random element from the array [3, 7, 9, 11]
console.log(sample([3, 7, 9, 11])); // Output: (random number from the array)
Output:
11
Visual Presentation:
Flowchart:
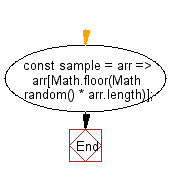
Live Demo:
See the Pen javascript-basic-exercise-149-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns a random element from an array using Math.random.
- Write a JavaScript function that selects an element at a random index from a given array.
- Write a JavaScript program that handles empty arrays gracefully when retrieving a random element.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to hash the input string into a whole number.
Next: Write a JavaScript program to run an given array of promises in series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.